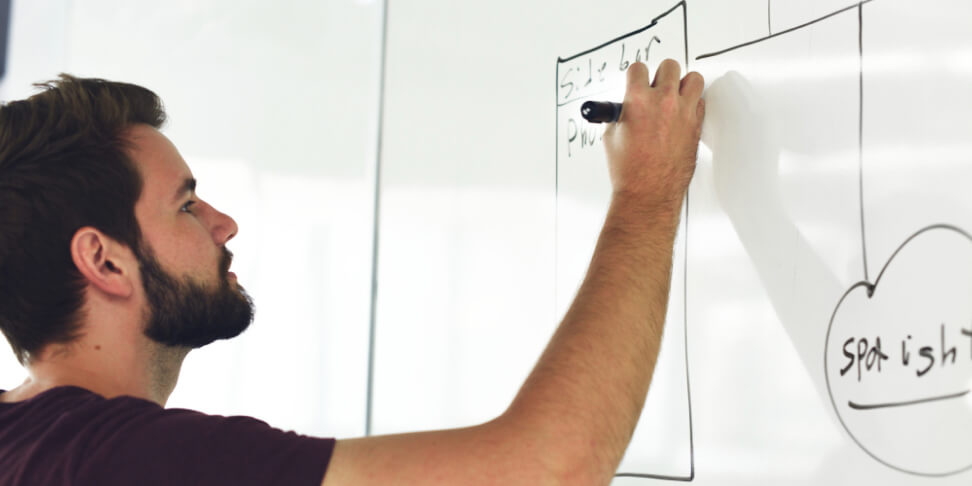
Autor: 17.03.2023
Algorithms - from block diagram to code
A large part of programming is writing various algorithms. How it works is - you get a block diagram of an algorithm and you have to make it into actual working code. That is a very important skill in every programmer’s toolkit.
In this article we will show you how to do just that! First we’ll try to analyze our algorithm, think of the consecutive steps and finally we will implement this block diagram as Python code.
What is an algorithm
The definition of algorithm is simple - it’s a set of actions leading to a certain result. It is a scheme of proceedings that need to be taken in order to resolve the problem.
An algorithm can be very simple and only involve a single operation. It could also be intricate and contain dozens of intermediate steps. In this article we have an algorithm that performs a check to see if the number given is positive.
The many ways of algorithm notation
Before we start writing any code, we should analyze our algorithm. Now, there are a lot of ways to note an algorithm down and present it.
You could, for example, write your algorithm as a list of steps. Basically you write, using clear and simple language, the consecutive steps of the algorithm in question:
1. Get a number
2. If the number is greater than 0:
a. Return True
3. Otherwise:
a. Return False
This simple method allows you to understand our algorithm easily, and make it into a piece of code.
Block diagrams
Oftentimes you will run into algorithms written down in the form of so-called block diagrams. They are sets of fields, shapes and arrows that visually represent what the algorithm does. Take a look at the diagram below:
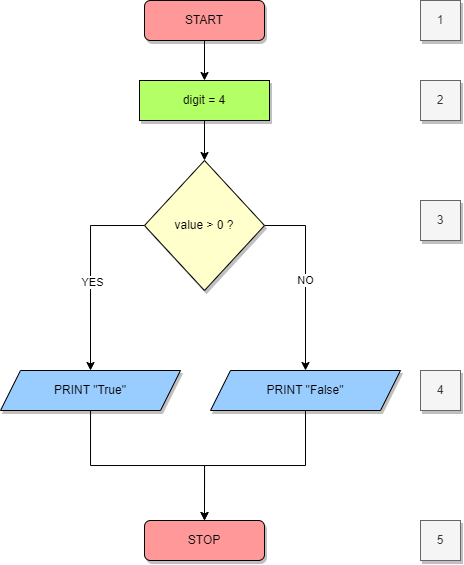
In the graph we can see our good pal, the algorithm checking whether a number is positive. Take notice of the numbers on the right side of the diagram’s blocks:
1. The START block that constitutes the beginning of the algorithm.
2. A block that performs an operation. In this case the operation is assigning a value to a variable.
3. A conditional block. In our algorithm’s case this is the value checking stage. We check whether or not the value is greater than 0 (positive) or not.
4. Two input/output boxes. They will be responsible for printing the data out. If the number is positive, we print out “True”, if not - “False”.
5. The STOP block that finishes the algorithm’s run.
In this stage we can already clearly tell what we are going to need, we know the steps of our algorithm. Nothing left but to turn it into code :)
If you want to draw your own block diagrams, you should take a peek into the free online tool that is diagrams.net. That’s also the tool we’ve used to make the graph above!
Translating a diagram into code
We will be working with Python in this one. Its clear and simple syntax will allow us to put the code down quickly - speaking of which, the code is really simple:
# 1
digit = 4 # 2
def is_positive(value):
if value > 0: # 3
return True
else:
return False
print(is_positive(digit)) # 4
# 5
First, we’re assigning a value to the digit variable (step 2). Next, we’re checking the value of the value parameter (step 3). Then, we’re calling our function inside a print() (step 4) and the result is as follows:
True
If the variable is equal 4, True is the returned result. As you can see, steps 1 and 5 were marked as comments in the code. They stand there for the START and STOP blocks, but in the actual code there’s no literal interpretation of that. We’d just added them to show you, as explicitly as possible, the translation of a graph to Python code.
Alternative methods
If you’re familiar with Python, you may have noticed that the code above could be shortened a lot. Like this, for example:
def is_positive(value):
return value > 0
print(is_positive(4))
Python allows you to write code in a very concise way, and so it’s worth using that quality of it. It’s still the same positive-number-checking algorithm as we’ve had before, of course.
The approach shown above is supposed to illustrate that block diagrams can be translated into code in many different ways. There’s no one correct solution. Our first presented approach better showed the method of translating a block diagram into code - the second one is more optimal, although less clear to a beginning programmer.
Summary
We hope this article does a good job of visualizing the thought process behind turning a block diagram into code. It’s one of the many essential for a programmer skills, and if you master splitting algorithms into consecutive steps, you will have no issue writing working code. You could even go as far as to say it’s the very basis of programming.
If you want to exercise your diagram-into-code skills and work on your algorithm writing skills, you can check out our course: Python - Practice Challenges.
There you will find 16 examples similar to the one we’ve worked with in this article. Your job will be to translate an algorithm into working Python code. It’s a great opportunity to exercise your coding and develop some problem-solving skills :)