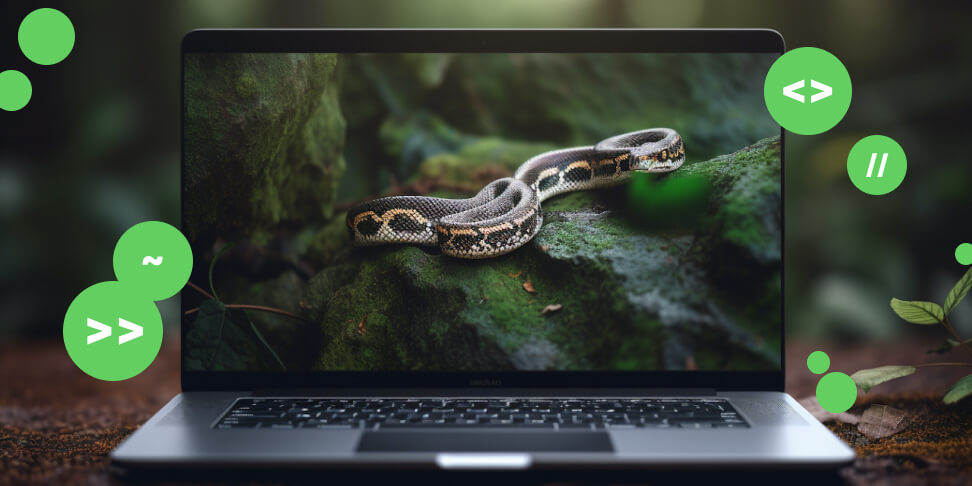
Autor: 21.06.2023
Python - simple tutorial for beginners
Python is a very popular language that is perfect for beginner programmers. It has a simple syntax and serves as a first step into the world of programming for many people. In this article we will show you how to write your first program in Python. Together, we will create a simple application that calculates the arithmetic mean of several numbers.
Our application is an excellent opportunity to demonstrate key concepts of the language. You will see that with just a few lines of code, you can create a simple and functional program.
What you will need
The code we will write needs to be executed - after all, we want to see its results. Python is an interpreted language, which means that we need a special program called an interpreter to run the code.
Fortunately, you don't need to install or configure anything. There are many online tools available where you can run your Python code. Here's an example of such a tool:
https://www.online-python.com/
Go to the above website. In the top part of the window, you can write your code. Below, there is a "Run" button that you can use to execute the code. The results will be visible in the console window at the bottom of the page.
Great! Let's now move on to the actual part of the article and start writing Python code.
Fundamentals of Python
At the beginning, we need to take a moment to discuss the most important concepts of the language. These are the fundamental building blocks that our program will be composed of. Let's get started!
Variables
Variables are a crucial part of almost every programming language. They allow us to store values, which can then be read and manipulated as needed. In Python, we define variables as follows:
sum = 0
We have created a variable named "sum" and assigned the value 0 using the equal sign. Now let's take a moment to think about what variables will be needed in our program for calculating the arithmetic mean.
- A variable to store the sum of numbers (which we already have).
- A list named "numbers" from which we will calculate the mean.
- A variable to store the result of the mean calculation.
So far, we have the "sum" variable, and we need to create the "numbers" list and another variable for storing the mean result.
Lists
Lists are variables that can store multiple values. Here's an example:
numbers = [5, 2, 8, 12, 3]
We have created a list named "numbers". Inside the square brackets, we added a list of numbers separated by commas. Our list contains five different numbers.
A for loop
We can use loops to repeat a certain action until a desired result is achieved. Here is an example of a loop in Python:
for number in numbers:
sum += number
Our loop processes each value from the "numbers" list sequentially until all the values have been exhausted. In other words, it continues until there are no more numbers in the list.
Each individual value from the list is represented by the variable named "number". Inside the loop, we perform an operation: we add each number to the "sum" variable we created earlier. This operation will be executed until all the numbers are summed. Why do we do this? Because before calculating the mean, we need to sum up all the numbers.
Important note! The operations inside the loop are indented. Notice that there are four spaces of indentation at the beginning of each line. This indentation is crucial because it tells the interpreter that these operations belong to the block of our "for" loop.
Operations
It's worth mentioning operations in this context. Inside the loop, we perform the summation using the following notation:
sum += number
In the above line of code, sum += number is a shorthand notation for adding the value of number to the current value of sum and updating the result back into the sum variable. It is equivalent to sum = sum + number.
This operation is repeated for each number in the list, accumulating the sum as we iterate through the loop.
In Python, you can use various arithmetic operators:
- "+" for addition,
- "-" for subtraction,
- "*" for multiplication,
- "/" for division.
These operators allow you to perform basic arithmetic operations on numbers in Python.
How to print a value
To make our program meaningful, it needs to output the calculation result to the screen. We can achieve this using the print statement:
print("Hello")
Inside the parentheses of the print function, you can provide the value that should be displayed on the screen. This can be a text string or the value of a variable.
Let’s build a final code
At this stage, you are familiar with the basic building blocks of a Python program. Let's recap: our goal is to write a program that calculates the arithmetic mean of given numbers.
Here is the final code for the program:
# Create a list of numbers
numbers = [5, 2, 8, 12, 3]
# Initilize a sum
sum = 0
# Calculate a sum
for number in numbers:
sum += number
# Calculate a mean
mean = sum / len(numbers)
# Print the result
print("Mena:", mean)
The only new element introduced in the final code is called comments. Comments are special code snippets that are ignored by the interpreter. Their purpose is to provide descriptions and explanations for individual lines of code in our program. Comments in Python start with the # symbol.
Here are the key elements of our program:
- First, we create a list named "numbers" that contains several integer values.
- We create a variable called "sum" with an initial value of 0.
- Using a for loop, we iterate through all the numbers in the list and add them to the "sum".
- We create a variable called "mean" and assign it the result of dividing the sum by the count of numbers because that's how we calculate the mean. We perform the division operation using the forward slash (/) operator.
- Using the print statement, we output the result to the screen, which is the value of the "mean" variable.
Notice that we use the function len(numbers) to check the length of the list of numbers. This allows us to know how many numbers are in our list. The len() function is built into the Python language, and you can use it whenever you need.
The len() function is a versatile tool that can be used with various data structures, including lists, strings, and tuples. It provides a convenient way to determine the length or size of these structures, which is often useful in many programming scenarios.
What can you do with the code
You can enter the code we provided into the online interpreter mentioned earlier to experiment with different sets of numbers. Simply modify the values in the numbers list to your desired input. For the numbers shown in the example, the mean is calculated to be 6.0.
Here's an example of how you can modify the numbers list:
numbers = [1, 3, 5, 7, 9]
In this case, the program will calculate the mean of the numbers [1, 3, 5, 7, 9], which should yield a different result than the previous example.
Feel free to explore and experiment with different values in the numbers list to see how the program calculates the mean for various sets of numbers.
Wrap up and next steps
We hope that this article has shown you how to take your first steps into the world of Python programming. In the beginning, it's important to master the fundamental building blocks and gradually start putting them together. Step by step, you can reach a decent level of proficiency. All it takes is a bit of enthusiasm and consistency in learning key programming techniques.
If you want to learn programming from scratch and acquire the necessary skills to work as a Junior Python Developer, check out the Python Developer Career Path. It offers dozens of examples similar to what we showed in the article. In addition to the absolute basics, you will also learn many practical working techniques.
Good luck on your journey!