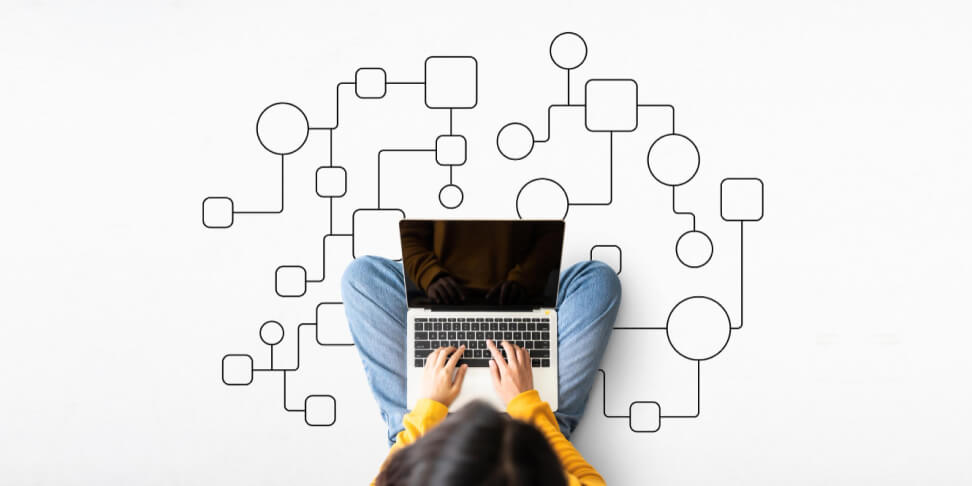
Autor: 28.04.2023
Algorithms in programming - different ways of notation
An algorithm, basically speaking, is a recipe for how to solve the problem at hand. It’s the game plan if you will, that will allow you to deal with the given task. Before we start the “dealing” in code though, it’s good to write it all out so it’s easier to visualize. That makes it more approachable, and helps you with analyzing and solving the issue.
In this article we will be talking about a few popular algorithm notations. Let’s get into it!
List of steps
Let's say we want to write a program that performs the simplest mathematical operation, that is: addition. The algorithm looks like this:
result = a + b
No surprise here, it is the simplest algorithm after all. Now we’ll try to write it down as a list of steps. Pretty simple, we’ll just list off all the actions needed in order to perform our algorithm. The list looks like so:
Step 1. Get a number.
Step 2. Get another number.
Step 3. Sum the two numbers.
Step 4. Show the result.
Step 5. End.
The list allows us to better understand our algorithm. Now we can easily tell what actions to perform to get the desired result. If you have an algorithm to write in code, try to do some analysis beforehand! Write down all the steps needed and you will have a much easier time working on the actual code.
Pseudocode
Another popular way of notation is the so-called pseudocode. Pseudocode is a fictional programming language that prioritizes readability over anything else. You don't have to stick to any particular rules or syntax, because pseudocode will not be run anyway - that’s not why it’s written. After you can clearly see your algorithm, you can write it out in your target language.
At this stage we focus on analyzing the operations necessary to perform our operation. In case of this particular algorithm, pseudocode could look like this:
begin
get(a)
get(b)
result:= a + b
write(result)
end
It’s a list of operations written in a fictional programming language. From here you can almost see the finish line :)
Block diagram
Another commonly used way to present an algorithm is a block diagram. You can imagine it as a set of blocks connected with arrows. The arrows specify how the information flows through our program, here’s an example:
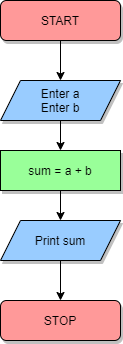
This of course is another rendition of the algorithm that adds two values together. In the diagram you can see, among other things, the starting block that, unsurprisingly, starts the algorithm’s run. Then there is the input block, which is responsible for getting our two values. The operation block, well, performs the operation of addition on the two numbers we got in the previous step. Next - the output block, which outputs our result. The whole thing ends at the STOP block.
In the article we’ve been using a simple example, but actual algorithms you might want to implement could be way more complex. The block diagram approach is pretty sensible because it allows you to see and analyze the relationships between all the steps in the algorithm. The arrows let you plan exactly the data flow in the program and everything looks more clear and readable.
If you're interested in creating your own block diagrams, it’s worth checking out the free online tool that is diagrams.net. This simple editor lets you create any and all sorts of diagrams for your algorithms.
Final code
Now, since we’ve talked so much about ways to write an algorithm down, how about we get to actual coding? We will implement the algorithm in Python:
a = 4
b = 2
sum = a + b
print(sum)
And that’s it in the code department! It’s a simple program that adds two numbers together, really as straightforward as you can get.
With an algorithm this simple we probably wouldn't be spending much time on the analysis stage. In practice though, our algorithms tend to be very much more complex than this. That is when the analysis comes in handy. And now, after reading this article, you know how you can go about it!
It’s not our first article on the important matter of algorithms - in another one, we have spoken about how to make a block diagram into code, and used a bit more complex of an example there.
And if you're really feeling this topic and want to go deeper into it, start with our Algorithms Level 1 course. There you will find a whole lot of examples that will make algorithm analysis and notation easier!