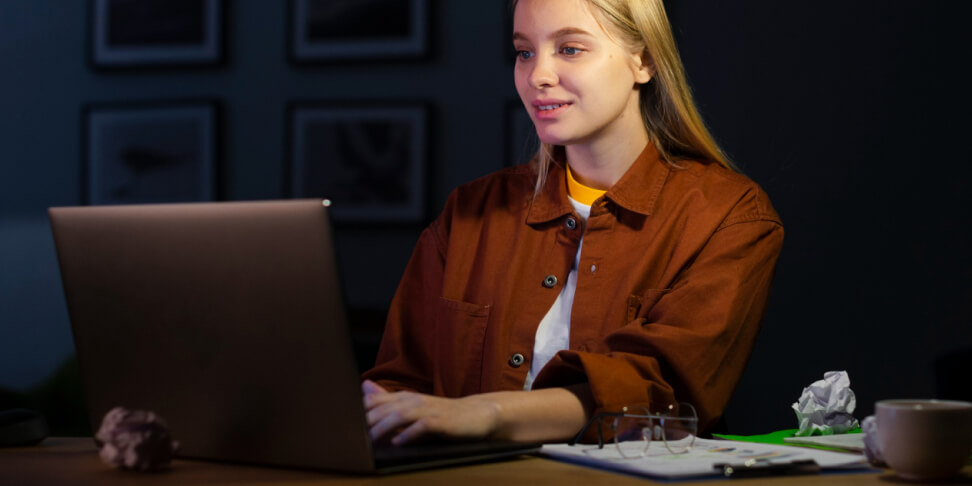
Autor: 16.01.2024
C - output and formatting
In the C language, we have a function that allows us to output data to the standard output, such as the console. We also have certain formatting options for the output. In this article, we will discuss the essential techniques related to using output in the C language.
Standard Output - How it Works
printf is the function that enables us to output data to the standard output. It is associated with modifiers that allow for data formatting. Look at how it works in practice:
#include <stdio.h>
int main() {
int a = 1;
int b = 2;
printf("Sum result: %d + %d = %d", a, b, a + b);
return 0;
}
The result of our program execution:
Sum result: 1 + 2 = 3
What really happened here? Those strange characters %d are called modifiers. The appropriate value is substituted for each modifier. How does it work?
- For the first occurrence of %d, we substitute the value of a, which is 1.
- For the second occurrence of %d, we substitute the value of b, which is 2.
- For the third occurrence of %d, we substitute the value of a + b (1 + 2), which is 3.
Formatting Different Data Types
Modifiers are associated with specific data types. In our example, the %d modifier is associated with int. Look at other examples:
TYPE | SPOKEN NAME | MODIFIER |
int | integer | %d |
long | long integer | %ld |
float | floating-point | %f |
double | double-precision | %lf |
char | character | %c |
There are more modifiers, but for now, this is sufficient. See how easily you can use these modifiers:
#include <stdio.h>
int main() {
int a = 1;
float b = 3.14;
char c = 'x';
long d = 123456;
double e = 123456.78;
printf("int: %d \n", a);
printf("float: %f \n", b);
printf("char: %c \n", c);
printf("long: %ld \n", d);
printf("double: %lf \n", e);
return 0;
}
As you can see, there's nothing particularly difficult here. For each type, we have a different modifier. In practice, it boils down to using the appropriate modifier.