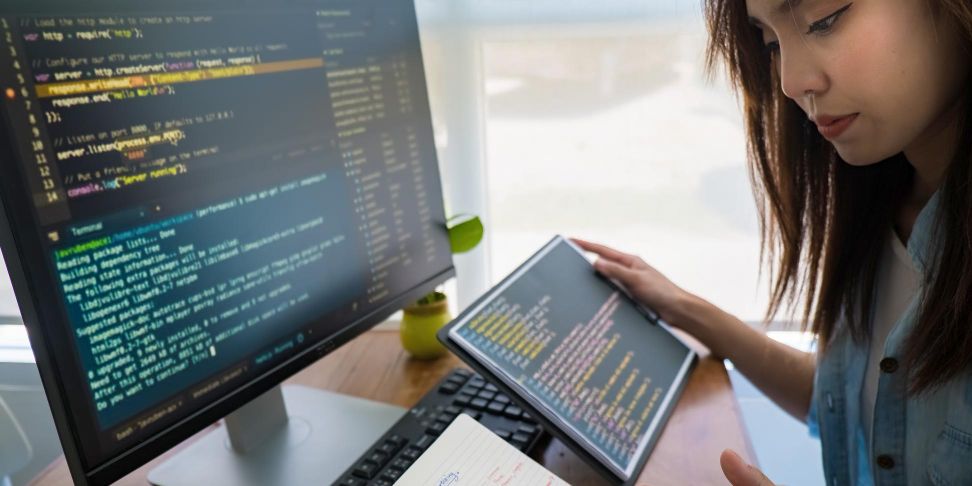
Article by: 20.07.2022
Clean Code - A practical introduction
Writing understable and clean code is a basic skill of every good Software Developer.
Programming almost always depends on a Teamwork. Every person in a team needs to follow the rules that were set by the team leader. One of the most important things is to write code with “Clean code” principles in mind. “Clean code” is a set of rules that tells us how to keep our code understable and easy to read.
Quality of your code tells a lot about your work attitude
One could write a really thick book about clean code. In practice it goes down to some rules that you should use on a daily basis. Learn them by heart, learn how to use them.
So let’s take a look at some of the most basic rules of a “Clean Code”. In the next part of the article you will see a few examples written in JavaScript. The language really doesn’t matter - what is really important is to understand the rules themselves. Think how you can implement them in your code.
Make the name of your variable easy to read and use
Let’s look at this variable:
var d = "Monday";
Its name is just a random letter without any logical meaning. To understand the purpose of the variable we need to look at its value. And that’s a pretty bad practice. We could really use a more meaningful name:
var dayOfWeek = "Monday";
Doesn't it look better this way? Of course it does! Now the name of the variable carries some meaning.
On the other hand, try to avoid unnecessary long names. Long names can sometimes result in an “information overload”. Reading code with very long names is tedious. Take a look at this example:
var userAccountList = [];
The name carries some meaning - it tells us a lot about the purpose of the variable. But it could be much shorter:
var accounts = [];
The accounts are associated with the users naturally. That's why we don’t need to state it explicitly. And the collection of accounts is…a list. And that’s another piece of information that doesn't have to be stated explicitly. Summing up - our short name ‘accounts’ combines all pieces of info into a single word. Looking at this name we know that this is a ‘list of user accounts’.
This area needs some balance. We want our names to be descriptive. On the other hand we want them to be short and concise.
Consequence is a key to writing quality code
Take a look at this code snippet:
const COLUMNS = 3;
const spacing = 20;
We got two constants. The name of the first one uses capital letters. And the name of the second one uses small letters. That’s simply a lack of consequence. The remedy for that is really simple:
const COLUMNS = 3;
const SPACING = 20;
Choose a single naming convention and stick to it. That way your code will be more readable.
Comments in code
Comments are useful but it’s really easy to fall into a trap. They are not a substitute for a quality code. Take a look at this example:
// calculate circle circumference
var r = 2.0; // radius
var result = 2 * 3.14 * r; // calculate circumference
console.log(result);
This part of the code calculates the circumference of the circle. Could it be done in a better way? Can we get rid of all those comments? Surely, we can:
var r = 2.0;
const PI = 3.14;
function calculateCircumference(radius) {
return 2 * PI * radius;
}
let result = calculateCircumference(r);
console.log(result);
INow the code is much, much better. We created a simple function with a meaningful name. The function takes a radius parameter that’s got a self-explanatory name. We also assigned a PI value to a constant with a meaningful name. That allowed us to get rid of all comments. The code is clean and self-explanatory.
Tip of the Iceberg.
In this article we showed you some examples of “Clean Code” rules. Keep in mind that this is only a short introduction to a much broader topic. Try to implement those rules in your code. Think of them every time you are coding. In the long run they will make you a better Software Developer.
If your brain is still hungry for knowledge in this topic, look at our course -Clean Code. It will guide you through the most important clean code rules. You will gain a lot of practical, applicable knowledge.