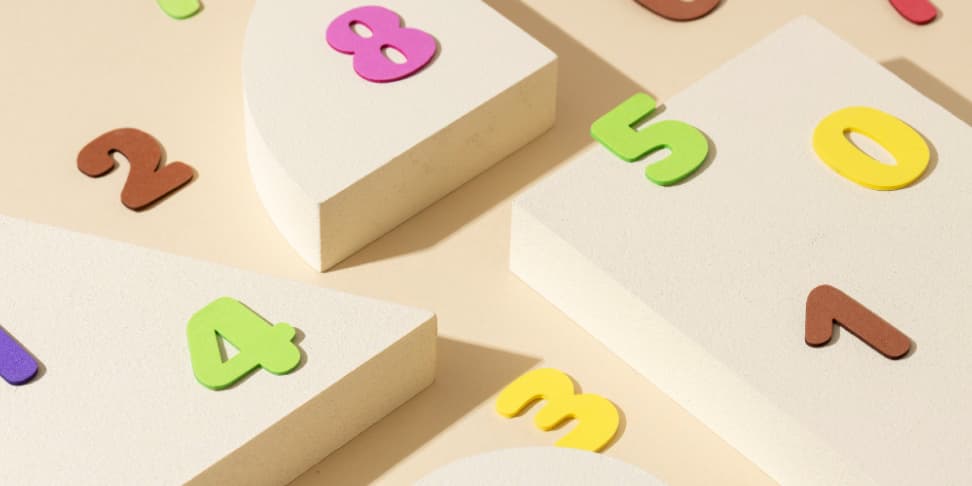
Autor: 23.02.2024
What Is The Difference Between Float and Integer?
Every programmer should know the difference between Integer and Float. Those two data types are the backbone of mathematical operations.
Integer
An integer (known also as int) is a whole number without a decimal part. It can be positive, negative, or zero. Examples of integers are -3, 0, 5, 100, and so on. The integer data type is used to represent values such as counting, indexing, or storing quantities that can only be whole numbers.
Float
Float (floating-point number) is a number that includes a decimal part. Examples of floating-point numbers are -3.14, 2.71828, 0.5, 1.0, and so on. The float data type is used to represent values that can have a decimal part or require high precision, such as measurements, calculations involving decimal values, or scientific computations.
Float vs. Integer: key differences
Integer | Float | |
---|---|---|
Representation | whole numbers | decimal component |
Precision | exact precision | limited precision |
Range | larger range of representable values | smaller range |
Memory Usage | less memory required | more memory reuired |
Mathematical Operations | basic mathematical operations such as addition, subtraction, and multiplication | complex mathematical operations involving decimal numbers, such as division, trigonometric functions, and logarithms. |
When choosing between float and integer, it is important to consider the nature of the data you are working with and the precision required for calculations. If you are dealing with whole numbers or require exact precision, use integers. If the data involves decimal parts or decimal calculations, use floats.
Examples in common programming languages
The topic of integers and floats interests us in the context of programming. Therefore, it is worth showing a few examples now.
Floats and Integers in Python
Here is a Python code snippet that demonstrates two variables, one of type integer and the other of type float. It is worth noting that in Python, you do not need to declare the variable type explicitly. The same variable can take values of both integer and float types.
integer_value = 10
float_value = 3.14
print(integer_value) # Output: 10
print(float_value) # Output: 3.14
Floats and Integers in Java
Java is another popular programming language. This time, we need to declare the variable type before assigning a value to it.
Here's an example in Java:
int integerValue = 5;
float floatValue = 2.718f;
System.out.println(integerValue); // Output: 5
System.out.println(floatValue); // Output: 2.718
Floats and Integers in C++
In C++, we have a similar situation to Java where we need to declare the appropriate type for variables.
Here's an example in C++:
int integerValue = 8;
float floatValue = 1.5;
cout << integerValue << endl; // Output: 8
cout << floatValue << endl; // Output: 1.5
Floats and Integers in JavaScript
Lastly, let's look at an example in JavaScript. We have dynamic types so it works just like in Python. The same variable can take both Float and Int types.
Here's an example in JavaScript:
var integerValue = 15;
var floatValue = 0.5;
console.log(integerValue); // Output: 15
console.log(floatValue); // Output: 0.5
Different languages, different implementations
Different programming languages may have different rules and behaviors regarding operations on floats and integers. It is always important to consult the documentation of the specific language to understand the details and any potential pitfalls.
Summary
Integer represents whole numbers without a decimal part, while float represents floating-point numbers with a decimal part. Integer has exact precision and a larger range, whereas float has limited precision and can represent numbers with a decimal part. These rules are universal and apply to most popular programming languages.