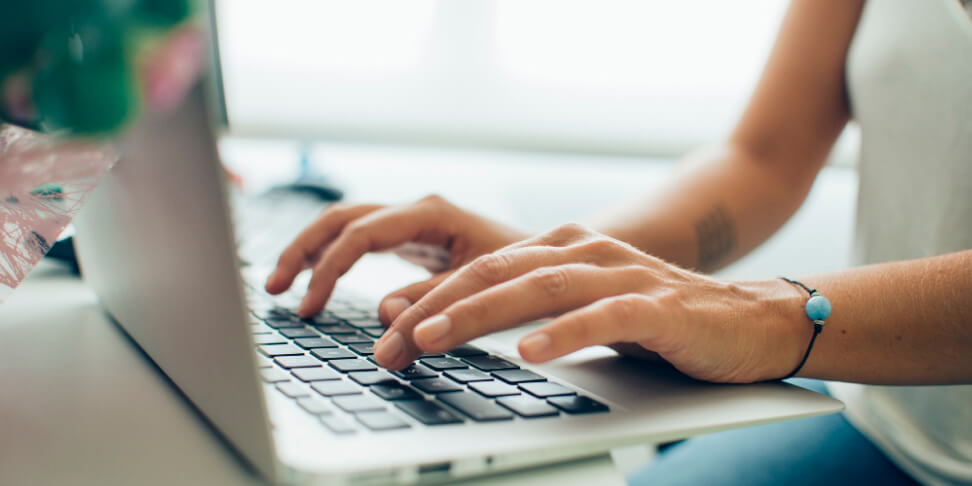
Autor: 26.06.2023
Haskell - how to declare and use functions
Haskell is a functional language. And functions are the basic building blocks of every app. This article will show you how to declare and use Haskell functions. Let’s start!
How to declare a function
In Haskell, a function is a separate piece of code that we can use multiple times. This prevents unnecessary duplication of our work.
Look at what a function declaration looks like:
add :: Integer -> (Integer -> Integer)
Strange symbols, aren't they? What is this all about?
Let's start at the beginning. We declare a function.
- It will be named add (don't forget the :: characters after the function name).
- It returns an Integer value.
- It will take 2 input parameters: (Integer -> Integer).
This is just a declaration. We are communicating that such a function will exist. We have not yet implemented exactly what it will do. We are only able to tell that such a function will deal with adding integers. Nothing else.
In this case, we will look at the definition of the add() function:
add digitX digitY = digitX + digitY
The example above is a definition. The add keyword is of course the name of our function. After the function name are the two input parameters. We have declared that there will be two parameters, so we have two. After the = sign we have the function body, which performs the appropriate actions. In our case the function had to deal with adding two integers, so it does it.
Let’s invoke our function
Once we have designed our function, we can now use it. We have prepared a declaration and definition. Now we have nothing else to do but to call it and test it in practice.
Calling a function involves using the name of our function and providing the appropriate arguments that we want to use for calculations. We want to add two integers 2 and 4:
print("Add two params: ")
let result = add 2 4
print(result)
We assign the result of our operation to the result variable. Our operation is the addition of two integers. After the = sign we call our function and we give the list of arguments: 2 and 4.
Here is the result:
"Add two params: "
6
Ultimately, our code should look like this:
add :: Integer -> (Integer -> Integer) -- declaration
add digitX digitY = digitX + digitY -- definition
main = do
print("Add two params: ")
let result = add 2 4 -- usage
print(result)
The add() function is called as follows:
let result = add 2 4
From the definition of the add() function, we can deduce that:
digitX = 2
digitY = 4
Putting the definition of the function under the formula, we have the following result of operations:
add digitX digitY = digitX + digitY
We provide two values:
add 2 4 = 2 + 4
The final result is:
add 2 4 = 6
Now that we've arrived at this result, let's take another look at the entire code:
add :: Integer -> (Integer -> Integer) -- declaration
add digitX digitY = digitX + digitY -- definition
main = do
print("Add two params: ")
let result = add 2 4 -- usage
print(result)
Now it is enough to substitute the digit 6 for add 2 4. The result of our calculations is identical to the result of the program:
6
Built-in functions
In the Haskell language, we have ready-made functions that we can use. Here are some functions with great possibilities.
In Haskell, there is a head() function that returns the first element of a list (head).
main = do
let list = [3, 1, 2]
let firstElement = head list
print firstElement
The head() function returns the first element of the list, so the result is as expected:
3
Another function which operates on lists is tail(). Its task is to search for the body of the list, that is, all the elements excluding the head (the first element). Look at the example:
main = do
let list = [3, 1, 2]
let body = tail list
print body
The result is in line with our expectations:
[1, 2]
The last() function, as its name suggests, will be able to return the last element of the list. Let's see how it works in practice.
main = do
let list = [3, 1, 2]
let lastElement = last list
print lastElement
Of course, the function works as intended:
2
More examples
Let's look at the table presenting other interesting functions operating on lists in Haskell.
Functions:
Init
Sample call | What does the function do? |
---|---|
main = print (init [1, 2, 3]) | Returns the elements of the list except the last one (reverse operation to tail()) |
Reverse
Sample call | What does the function do? |
---|---|
main = print (reverse [1, 2, 3]) | Reverses the order of the list elements. The last element becomes the first element, and so on. |
Length
Sample call | What does the function do? |
---|---|
main = print (length [1, 2, 3]) | Returns the number of elements in the list. |
Maximum
Sample call | What does the function do? |
---|---|
main = print (maximum [1, 2, 3]) | Returns the largest element of the list. |
Minimum
Sample call | What does the function do? |
---|---|
main = print (minimum [1, 2, 3]) | Returns the smallest element of the list. |
Sum
Sample call | What does the function do? |
---|---|
main = print (sum [1, 2, 3]) | Sums the elements of the list. |
The features we have presented are just a small taste. The language offers much more, but we are not able to show everything in this short article.
Summary
We hope that you learned something about Haskell functions. They are the basic building block of almost every program written in this language.
Wanna learn more about Haskell functions? Start the free course Haskell Fundamentals!