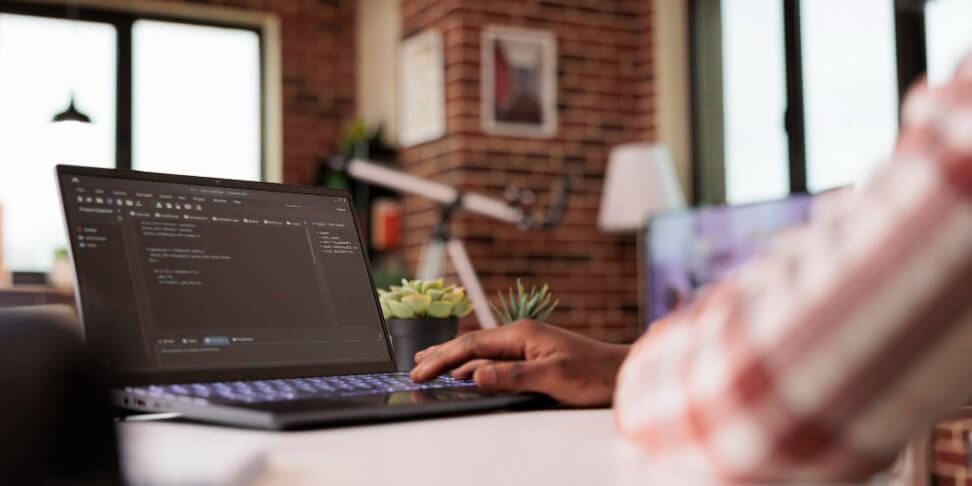
Autor: 19.05.2023
How to use PHP functions - a simple guide
Functions are a crucial part of most programming languages. In this article, you will learn key techniques for working with functions in PHP. We will start with the basics and then move on to several practical working techniques.
Function arguments
Let's refresh our memory on how functions work in PHP. Let's jump straight into an example:
function sum($a, $b) {
echo $a + $b;
}
- Here, we have defined a function named sum() that takes two parameters: $a and $b.
- The function uses echo to output the sum of $a + $b.
Here is a key thing:
sum(2, 3)
During the function call, you need to provide values for $a and $b. In this example, we will obtain a result (sum) equal to 5.
5
Function returning a value
Most functions in PHP return a value, allowing us to do more than just output it to the browser. We can use the returned value in any part of the program for various purposes.
function multiply($a, $b) {
return $a * $b;
}
Here we have the multiply() function, which multiplies the values of the $a and $b parameters. It includes a return statement, which specifies the value to be returned by the function. This value will be the result of the multiplication.
Now you can assign the result of the function to a variable:
$result = multiply(2, 4);
Now, the variable $result stores the multiplication result returned by the multiply() function.
Default arguments
Sometimes, we may want to create default parameters for functions. Omitting certain parameters can be desirable in certain situations. Take a look at the following example:
<?php
function printLine($size, $sign) {
for($i = $size; $i > 0; $i--) {
echo $sign;
}
echo "\n";
}
printLine(5, '*');
printLine(4, '*');
printLine(3, '*');
?>
And here is the result:
*****
****
***
You can optimize this task. Notice that we are unnecessarily calling the function multiple times with the asterisk (*) parameter. You can introduce a default parameter in the function like this:
<?php
function printLine($size, $sign = '*') {
for($i = $size; $i > 0; $i--) {
echo $sign;
}
echo "\n";
}
printLine(5);
printLine(4);
printLine(3);
?>
The program's outcome is identical to the previously presented example. Take a look at how, with a small effort, you can save a bit of typing. The result:
*****
****
***
If you don't provide the second parameter in the function call, the value of the $sign parameter will take the default value assigned to that parameter, which is '*'.
When you start working with default arguments in functions, you need to keep a few aspects in mind:
If you specify a default value for an argument in the function declaration, you must provide default values for all subsequent arguments in the list.
<?php
// incorrect
function printLine1($a, $b = 1, $c) { }
function printLine2($a = 1, $b, $c) { }
//correct
function printLine3($a, $b, $c = 2) { }
function printLine4($a, $b = 1, $c = 2) { }
?>
If a function has multiple default parameters, when omitting one of them, you must also omit the subsequent ones:
<?php
function printLine($a, $b = 100, $c = 200, $d = 300) { }
// correct
printLine(10);
printLine(10, 1);
printLine(10, 1, 2);
printLine(10, 1, 2, 3);
// incorrect
printLine(10, , 2);
printLine(10, , , 3);
?>
You cannot omit parameters. If you choose to omit one default argument, then you must omit the subsequent parameters and rely on their default values.
Passing the arguments by value and by reference
Pass by value
The following example illustrates how you can pass a parameter by value to a function:
<?php
function showValue($digit) {
$digit = 14;
echo "A variable inside function: $digit \n";
}
$digit = 17;
echo "A variable before the function call: $digit \n";
showValue($digit);
echo "A variable after the function call: $digit \n";
?>
And here is the result:
A variable before the function call: 17
A variable inside function: 14
A variable after the function call: 17
We change the value of the variable inside the function, but in the end, its value remains 17. This happens because the parameter in the function is actually being copied, and its lifespan is tied to the duration of the function. The variable ceases to exist at the end of the function block.
Pass by reference
Let's now add a bit of programming magic.. Not much will actually change, but you'll see the tremendous consequences that come with passing parameters by reference.
<?php
function showValue(&$digit) {
$digit = 14;
echo "A variable inside function: $digit \n";
}
$digit = 17;
echo "A variable before the function call: $digit \n";
showValue($digit);
echo "A variable after the function call: $digit \n";
?>
The result will be completely different:
A variable before the function call: 17
A variable inside function: 14
A variable after the function call: 14
The program itself hasn't changed. Only the way the parameter is passed to the function has changed. Notice that there is a special symbol, the ampersand (&), before the parameter. This symbol ensures that our parameter is not copied but passed to the function as the original. By making changes within the function, you modify the original value. Hence, the result is as observed, and not otherwise.
Variable number of arguments
Sometimes you may encounter a situation where you are unsure about the number of arguments you can pass to a function. In such cases, the so-called splat operator comes to the rescue.
You can recognize the splat operator by the distinctive "..." appearing before the parameter name in a function. This operator allows us to pass any number of arguments. Remember that the splat operator can only be used as the last parameter of a function.
<?php
function printData(...$values) {
foreach($values as $value) {
echo "$value \n";
}
}
printData(1,2);
printData("Tom", "John", "Mike");
?>
Take a look at the parameter of the function printData(). This peculiar construction is called the splat operator. Thanks to this specific operator, we can pass an unspecified number of parameters (in other words, any number) to the function.
And here is the result:
1
2
Tom
John
Mike
The declaration of such a parameter must be done in a certain way. Now we will present a correct and incorrect declaration.
Incorrect:
<?php
function printData(...$values, $digit) { }
?>
Correct:
<?php
function printData(...$values) { }
function printData($digit, ...$values) { }
?>
An argument (parameter) accepting an arbitrary number of values must be placed at the end of the function's parameter list. This applies when the function has more than one parameter.
Summary
Functions are a very important part of PHP programming. They allow us to reuse the same block of code multiple times. In this article, we have shown key techniques for working with functions.
If you want to learn more about functions and beyond, start the PHP Developer Career Path. With this path, you will learn PHP language from the basics to an intermediate-advanced level. The path concludes with an Exam that qualifies you to obtain the Specialist Certificate.