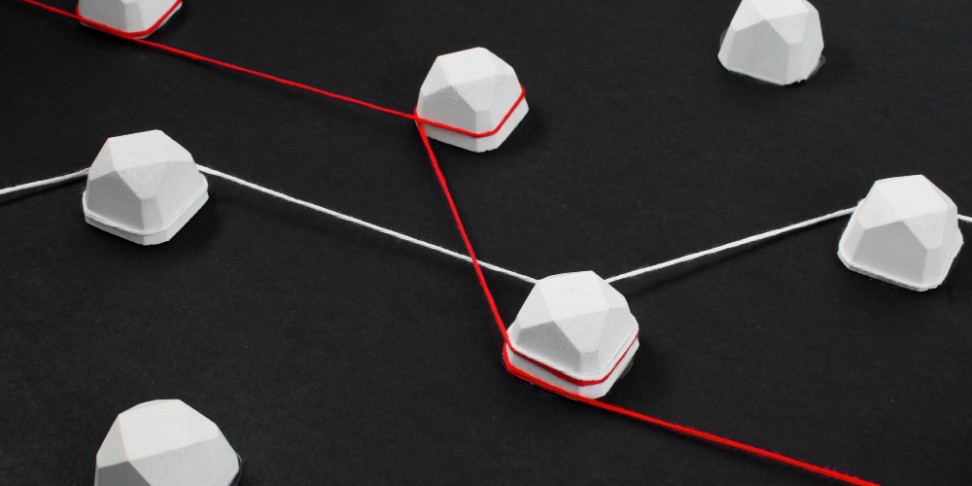
Autor: 12.05.2023
How to use Structures (Struct) in C
Structures (Structs) are a great way to group related information together. If you have a set of variables in your code that store logically connected data, consider combining them into a single entity - that is, a Structure. In this article, you will learn the most important techniques for using Structures in the C language.
A basic Structure
Let’s start with a simple example:
#include
struct Car {
char *mark;
int productionYear
int maxSpeed;
};
int main() {
return 0;
}
Pay attention to the keyword "struct". Our variables describing a mechanical vehicle (i.e., Car) should be enclosed in curly braces, and the entire structure should be terminated with a semicolon. Inside the structure, there are variables of various types: integer types (int) and a pointer (char*). This will be our very basic structure, grouping information about a car.
Access the values
You need to imagine that a structure is actually a new data type. In the example below, we will show you how to define types for structures and how to set values:
#include
struct Car {
int productionYear;
int maxSpeed;
};
int main() {
struct Car audi;
audi.productionYear = 2017;
audi.maxSpeed = 200;
printf("audi production year: %d \n", audi.productionYear);
printf("audi max speed: %d \n", audi.maxSpeed);
return 0;
}
And here is the result:
audi production year: 2017
audi max speed: 200
Let's analyze what happened in the main() function. At the beginning, we define a variable named "audi" which is of type struct Car. With the variable "audi," we can modify the elements of the structure. To access a structure element, we use the dot operator:
audi.productionYear = 2017;
So we got this simple syntax:
[ name of the struct variable ] [ . ] [ name of the value ] = [ value ] [ ; ]
As you can see, there's nothing difficult here. A structure (Struct) is simply a set of related data. Access to a field within a structure can be obtained using the dot operator.
Structures and functions
Now it's time for a more advanced technique. Sometimes there is a need to pass a structure to a function. Passing a structure to a function involves making a copy of it and passing it as an argument. Here's a code snippet to demonstrate this:
#include
#include
struct Person {
char name[20];
int age;
};
void displayPerson(struct Person person) {
printf("Name: %s\n", person.name);
printf("Age: %d\n", person.age);
}
int main() {
struct Person p1;
strcpy(p1.name, "John");
p1.age = 30;
displayPerson(p1);
return 0;
}
In this example, the structure "Person" is passed to the function "displayPerson()" as an argument by value, which means it is copied into a local variable "person" (the function parameter). Inside the function, the fields "name" and "age" can be accessed using the dot operator ".".
It's important to note that for large structures, passing them by value can be inefficient in terms of memory consumption and time. In such cases, it is recommended to use pointers.
And here is the result:
Name: John
Age: 30
You already know another useful technique for working with structures. It's time for the next example. Our previous function had the ability to print information from a structure. Of course, there is nothing stopping us from returning a structure from a function. Here is an example that demonstrates such behavior.
#include
#include
struct Person {
char name[20];
int age;
};
struct Person updatePerson(struct Person person) {
strcpy(person.name, "Bob");
person.age = 30;
return person;
}
int main() {
struct Person p1;
strcpy(p1.name, "Alice");
p1.age = 25;
struct Person p2 = updatePerson(p1);
printf("Name: %s\n", p2.name);
printf("Age: %d\n", p2.age);
return 0;
}
In this example, the function "updatePerson()" takes the structure "Person" as an argument, named "person" (by value). It modifies the fields of the structure and then returns the modified structure as the return value.
In the "main()" function, we initialize a structure "p1" and then call the "updatePerson()" function, passing "p1" as an argument. After calling the function, we read the fields of the returned structure "p2" and display them on the screen.
The result is as follows:
Name: Bob
Age: 30
These examples illustrate the potential that lies in using structures and functions. As you can see, structures can be passed as arguments and can also be returned by functions.
Structures and pointers
Pointers are an essential part of the C language, and they can also be used to work with structures. Take a look at the example below:
#include
struct Point {
int x;
int y;
};
int main() {
struct Point point;
struct Point* pointer = &point;
pointer->x = 5;
pointer->y = 7;
printf("x = %d, y = %d \n", pointer->x, pointer->y);
return 0;
}
And here is the result:
x = 5, y = 7
In the above code, we have a crucial line of code where the pointer "ptr" is assigned the address of the "point" object:
struct Point* pointer = &point;
After such an assignment, using the pointer "ptr", we can access the fields of the structure using the "->" operator:
pointer->x = 5;
Summary
Structures allow you to easily group related data together. You can pass them as parameters to functions, return them from functions, create pointers to structures, and more. With structures, you can achieve clean and organized code where data is logically grouped together.
If you want to master Structures and many other practical techniques in C, enlist for the C Developer Career Path. With this career path, you will learn the C language from the basics to an intermediate-advanced level. The path concludes with an exam that grants you the Specialist Certificate, enabling you to demonstrate your proficiency with the C language.