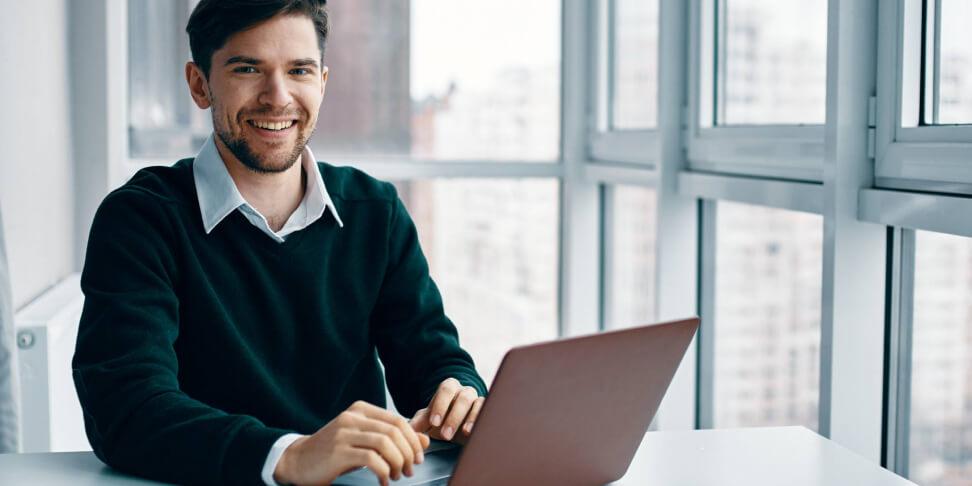
Author: 24.03.2022
What is this all about?
Programming requires the right approach. Every program is different. Every program needs to be “figured out” in the right way.
This is why there are so-called programming paradigms. They are nothing more than a certain style of writing code. In this article, we will take a closer look at the two main programming paradigms.
Imperative programming
The imperative paradigm can be described like this: "we describe how a computer should do a given thing". In other words, we focus on the individual steps that lead to the solution of the problem.
You can picture a program written imperatively as a sequence of instructions. This approach is easy for us to understand. It is easy to keep track of all the steps performed by the program. For this reason, imperative programming is usually the first paradigm that novice programmers encounter.
Example
This can best be explained with an example based on real code. We have a simple array of numeric values and we want to discard values equal to 1 from it. Look at the code in JavaScript:
let arr = [1, 2, 3];
let filteredArr = [];
for (let i = 0; i < arr.length; i++) {
let v = arr[i];
if (v != 1) {
filteredArr.push(v);
}
}
console.log(filteredArr);
This is, of course, an example of code written imperatively. Notice that we have to describe step by step exactly all the instructions that are to be executed:
- Create a new, empty array named filteredArr.
- Use the loop to process the arr array. End the loop when all the values in the array are used up.
- In the loop, create a variable v that stores individual values from the array.
- Use the conditional statement to check the value of the v variable.
- If the value of v is different than 1, insert such a value into the filteredArr array.
Here we simply "hold the computer's hand" and tell it exactly what to do in each step.
Declarative programming
The declarative paradigm can be described like this: "we tell the computer what to do for us". What is most important for us is the result we obtain - we do not care about how the computer achieves this result.
So this style of programming allows us to focus on the goal. On what we want to achieve. Less important here are the steps that lead to this goal.
Example
We will now try to solve the familiar problem in a more declarative way:
let arr = [1, 2, 3];
function checkValue(v) {
return v != 1;
}
console.log(arr.filter(checkValue));
The goal is the same as before: we want to discard values equal to 1 from the arr array. Note the major components:
- We wrote a simple function that checks the value and returns true if the condition is met.
- We use a built-in JavaScript function called filter(). Using it we can filter values from the array. As a parameter, we have given our function which verifies if the value is different from 1.
The important thing is that we do not go into the details of the filter() function. We are not interested in what it contains "under the hood". The important thing is that the function will give us the desired result.
Note that this style of writing code is meant to be concise - usually declarative code is shorter and more elegant than imperative code.
Pros and cons
Both paradigms have their pros and cons, of course.
Imperative programming
- It is simpler to understand for beginners.
- We can easily follow all the steps.
- Requires writing more complex code. There may be problems with readability, especially for larger programs.
Declarative Programming.
- Code is more concise and readable.
- It allows us to focus on the result and not on the way we solve the problem. This is a very important aspect.
- It requires more experience and good knowledge of the mechanisms of the language in which we work.
The main advantage of the declarative approach is that we focus on the outcome we want to achieve. This allows us to shift our thinking to the right track. We don't get into single steps but we think about the result all the time - it is the key here. In practice, this allows us to write more concise and easier to maintain code.