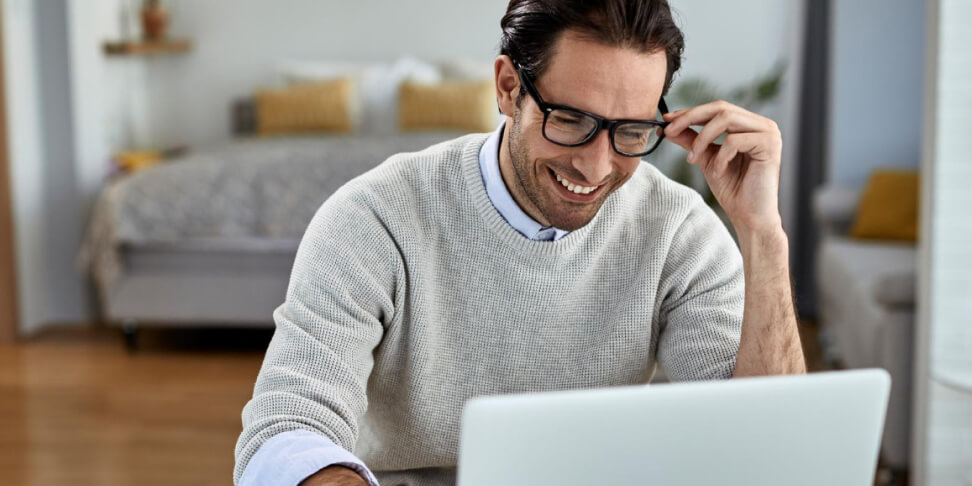
Autor: 18.03.2024
Input and Output Operations in Programming
Input and Output (I/O) operations are the way programs communicate with users and the external environment. They are a crucial element in every program. For beginner programmers, understanding the basic concepts and practical application of input and output operations is extremely important.
Input and Output in Programming
Input refers to any data that the program receives from the user or another external source, such as text files, data from a database, etc. Output is the data generated by the program and passed to the user or another system, such as calculation results, user messages, file writing, etc.
Input and Output Operations in Python
In Python, input and output operations are performed using built-in functions and objects.
User Input
To obtain data from the user, we can use the input() function. For example:
name = input("Enter your name: ")
print("Hello,", name)
The input() function returns the text entered by the user, which we can assign to a variable.
Output for the User
To display messages for the user, we use the print() function. For example:
File Operations
Python also allows working with files. We can open, read, write, and close files using built-in functions. For example, to read the contents of a text file:
with open("file.txt", "r") as f:
content = f.read()
print(content)
Input/Output Operations for Files
We can also read and write data to files using input and output operations. For example, to write something to a file:
with open("results.txt", "w") as f:
f.write("This is some text.")
Summary
Input and output operations are a crucial element of every program. In Python, we use the input() function to get data from the user, the print() function to display messages, and file operations to work with data saved on the disk.