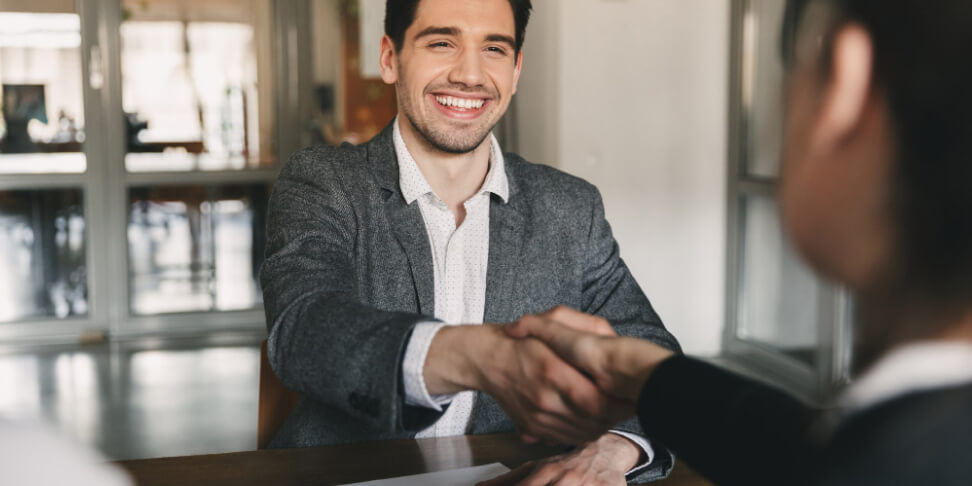
Autor: 01.08.2023
Java - example interview questions
Every job interview is stressful, especially the ones that require extensive skills and a good understanding of the subject matter. In this article, we will show you five sample interview questions for the position of Junior Java Developer.
What are interfaces in Java?
Interfaces in Java are one of the key elements of object-oriented programming. They allow the definition of contracts that classes implementing the interface must fulfill.
An example of interface usage could be creating a Shape interface that defines methods related to shapes. The Circle and Rectangle classes implement this interface, which means they have to implement all the methods defined in the Shape interface.
interface Shape {
double area();
}
class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double area() {
return Math.PI * radius * radius;
}
}
class Main {
public static void main(String[] args) {
Shape circle = new Circle(2.0);
System.out.println(circle.area());
}
}
The Shape interface defines the area() method. The Circle class implements this interface, which means it must implement the area() method. This allows us to invoke the method on Circle objects regardless of their specific implementations.
Here is the result of the above code:
12.566370614359172
What are exceptions in Java?
The exception mechanism in the Java language allows for easy and safe handling of errors in a program. When an error occurs, the program can throw an exception, which will be caught and handled appropriately.
An example of using the exception mechanism is division by zero. In such a situation, the program can throw an ArithmeticException, which will be caught and handled accordingly.
public class ExceptionExample {
public static void main(String[] args) {
try {
int result = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("Exception!");
}
}
}
In the above example, the program attempts to divide the number 5 by 0, which results in an error. When the error occurs, the program throws an ArithmeticException, which is caught in a try-catch block. In the catch block, the exception is handled, and the message "Exception!" is printed.
Here is the result of the above code:
Exception!
Explain the difference between ‘text’ and ‘new String(“text”)’
Take a look at this code snippet:
String text1 = "text";
String text2 = new String("text");
The variable text1 is of type String and contains the value "text". The variable text2 is also of type String but was created using the new String() constructor. This constructor creates a new String object based on the argument passed to it, which in this case is "text". This means that the variable text2 refers to a new String object that has the same value as the String object representing "text" created in memory.
We can verify if text1 and text2 are different objects with identical string values.
System.out.println(text1 == text2); // false
System.out.println(text1.equals(text2)); // true
In the first call to the println() method, we are comparing the references to two String objects - text1 and text2. Since text2 was created using the new String() constructor, it refers to a new String object that is different from the object referred to by text1. Therefore, the result of the reference comparison is false.
In the second call to the println() method, we are comparing the values stored in text1 and text2 objects. Both variables hold the string "text", so the result of the value comparison is true.
What’s the difference between ArrayList and LinkedList?
In Java, there are two popular implementations of the list: ArrayList and LinkedList. Both implementations allow storing elements in a specific order but differ in their storage and access methods.
ArrayList is an implementation of a list based on an array. This means that elements are stored in the order they were added, and each element is stored in a specific position in the array. ArrayList provides fast access to elements based on their index, which means accessing an element at a specific index is efficient. However, adding or removing elements from the middle of an ArrayList is costly because it requires shifting all elements by one index.
ArrayList arrayList = new ArrayList();
arrayList.add("element 1"); // add element
arrayList.add("element 2");
arrayList.add("element 3");
String element = arrayList.get(1); // get element
arrayList.remove(0); // remove element
LinkedList is an implementation of a list based on nodes. This means that each element is stored in a separate node, and each node contains references to the next and previous nodes in the list. As a result, adding or removing elements in the middle of the list is efficient because it only requires changing the references to neighboring nodes. However, accessing an element based on an index is costly because it requires traversing the entire list.
LinkedList linkedList = new LinkedList();
linkedList.add("element 1"); // add element
linkedList.add("element 2");
linkedList.add("element 3");
String element = linkedList.get(1); // get element
linkedList.remove(0); // remove element
In summary, ArrayList provides fast access to elements based on their index, but adding or removing elements in the middle of the list is costly. LinkedList, on the other hand, offers efficient insertion and removal of elements in the middle of the list, but accessing an element based on an index is costly. The choice between ArrayList and LinkedList depends on the specific project requirements and the nature of the operations performed on the list.
What is an anonymous class in Java?
An anonymous class in Java is a class that has no name and is defined at the point of use. It is useful when we want to define an implementation of an interface or an extension of an abstract class in one place without creating a separate class.
Here is an example:
interface MyInterface {
void doSomething();
}
public class Main {
public static void main(String[] args) {
MyInterface myObject = new MyInterface() {
@Override
public void doSomething() {
System.out.println("I work!");
}
};
myObject.doSomething();
}
}
And here is the result:
I work!
In the above example, we define an anonymous class that implements the MyInterface interface. This class has no name but contains an implementation of the doSomething() method. Then, we create an object of this anonymous class and invoke its method.
An anonymous class is created at the point of use, so we cannot refer to it outside that location. It is useful for simple implementations that are needed only in one place and do not require a separate class.
Summary and next steps
The questions in this article are intended to show you what you can expect in a job interview. Treat them as a guideline for the expected level of your skills.