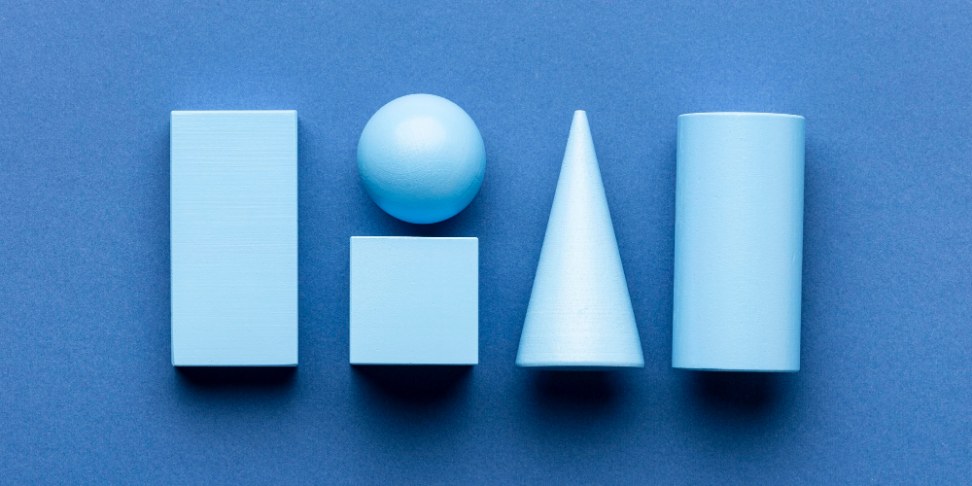
Autor: 24.05.2023
OOP in C++ - a very basic guide
Classes and objects
As you probably know, in the C++ language, we have different data types such as string, int, bool, and so on. These are examples of built-in types that are readily available. You also have the ability to create your own types.
A class is your own type. Classes were invented to bring certain objects from the real world into our code. Just like certain objects in the real world, our classes have specific behaviors and properties. For example, a "washing machine" object can wash, rinse, spin, and so on.
Take a look at this simple example of a class:
class WashingMachine {
};
To define a class, you need to use the keyword "class," followed by giving a name to your class. The body of the class is enclosed in curly braces { and }. Remember that we end the class definition with a semicolon ";".
A class is literally a type. Now, let's define our own variable using "int" and the class "WashingMachine":
int operation;
WashingMachine amica;
The above example illustrates the usage of variables of types "int" and "WashingMachine". The variable named "amica" is a specific object of the "WashingMachine" class.
What’s the difference between a class and an object?
The class definition serves as a template in a factory, while an object is the finished product that comes off the production line. In our case, it would be the amica washing machine, which is of type WashingMachine. There is nothing preventing us from creating an object with a different name but the same type. For example:
WashingMachine whirlpool;
Fields
To make a class more interactive, we can add components to it (fields). These components can be variables that are included in our class. Let's analyze the code below, as it contains several interesting pieces of information.
class WashingMachine {
public:
int programNumber;
int size;
private:
float engineSpeed;
protected:
string name;
};
The code presents the WashingMachine class, which includes fields such as programNumber, size, engineSpeed, and name. Class fields are nothing more than regular variables. We can set the values of class fields, as you will see in a moment.
Access modifiers
It can be noticed that the code includes labels: public, private, and protected. They are not mandatory - if not specified in the class, the fields are by default considered private. What is the purpose of these labels? They allow us to restrict or relax access to class fields. What levels of access do the labels represent?
- Public: Members (fields and functions) labeled as public are accessible from anywhere in the program. They can be accessed by objects of the class, as well as from outside the class.
- Private: Members labeled as private are only accessible within the class itself. They cannot be accessed by objects of the class or from outside the class. Private members are typically used for internal implementation details and are not directly accessible to external code.
- Protected: Members labeled as protected are similar to private members, but they are also accessible by derived classes (classes that inherit from the base class). Protected members can be accessed within the class itself, as well as by derived classes.
By using these access labels, we can control the visibility and accessibility of class members, ensuring encapsulation and data hiding principles.
How to access class fields
Take a look at this piece of code:
#include <iostream>
using namespace std;
class WashingMachine {
public:
int size;
};
int main() {
WashingMachine beko; // object
WashingMachine *wsk; // pointer
WashingMachine &ref = beko; // reference
beko.size = 50;
wsk->size = 50;
ref.size = 50;
cout << beko.size << endl;
cout << wsk->size << endl;
cout << ref.size << endl;
return 0;
}
Access to public class member fields is realized in the following way:
How to object is declared | How the field is accessed |
---|---|
Object (beko) | Dot - beko.size |
Pointer (*wsk) | Arrow - wsk->size |
Reference (&ref) | Dow - ref.size |
Constructor
A very important role in object-oriented programming is played by the so-called constructor. The constructor has several characteristics that can be observed in the code. The constructor does not return any value and has the same name as the class in which it appears. Here is an example of such a constructor:
#include <iostream>
using namespace std;
class Car {
string name;
public:
Car(string mark) {
name = mark;
cout << "Constructor is working, setting: " << name << endl;
}
};
int main() {
Car audi = Car("audi");
return 0;
}
Note that the "name" field is private, and the constructor is public. The constructor can operate on private fields, and it does so by setting the class member
Constructor is working, setting: audi
Functions (Methods)
The member function of the Car class has been presented in the code below. A member function has scope throughout the class and has access to its members, allowing it to operate on them. Let's take a look at the definition of the method and its usage:
#include <iostream>
using namespace std;
class Car {
private:
string name;
public:
Car(string mark) {
name = mark;
}
string getName() {
return name;
}
};
int main() {
Car audi = Car("audi");
cout << audi.getName() << endl;
return 0;
}
Using the constructor, we set the brand of the car. The getName() method can operate on the member fields of the Car class. The method is public, so we can invoke it from outside. The result of our code looks like this:
audi
Summary
In the article, we have presented key concepts related to object-oriented programming in the C++ language. This is just the beginning of the possibilities offered by this language - the topic of object-oriented programming is very broad. We have provided a starting point with this short article.
If you want to learn C++ from scratch and acquire the skills needed for a Junior C++ Developer position, you can start learning with the C Developer Career Path. The learning path concludes with an exam that qualifies you to use the Certificate of Completion.