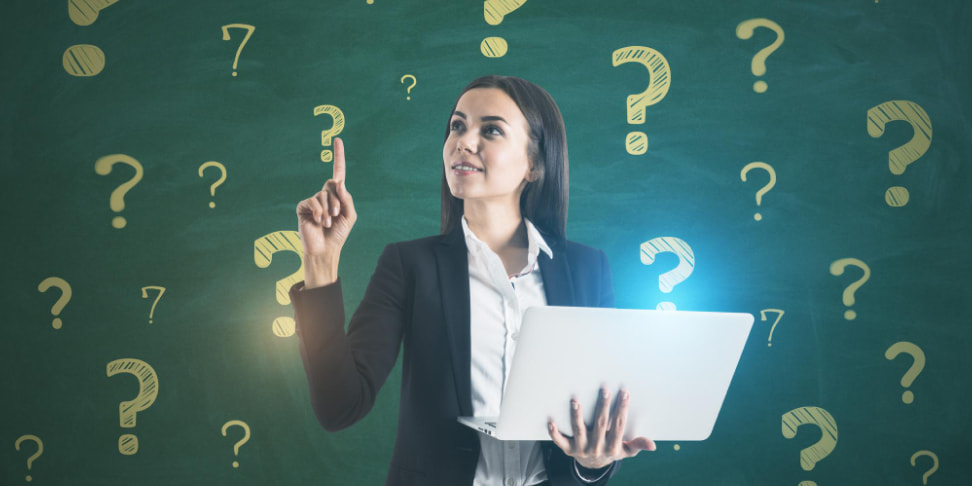
Autor: 29.09.2023
PHP - Sample Interview Questions
Test your knowledge of the PHP language. We have prepared 10 sample questions that often come up in recruitment interviews. They cover a wide range of PHP basics concepts.
10 PHP Questions - Test Your Skills
Question 1
How do you add a new element to the end of an array in PHP?
A) array_unshift()
B) array_push()
C) array_shift()
D) array_pop()
Correct answer: B. You can use the built-in function array_push() to add an element to the end of an array.
Question 2
What will be the result of executing the following function in PHP?
function addNumbers($num1, $num2 = 10) {
return $num1 + $num2;
}
echo addNumbers(5);
A) 5
B) 10
C) 15
D) Error
Correct answer: C. This is an example of a function with a default value for the $num2 parameter (which is 10). We provided a value only for the first parameter, so the second one will have the default value.
Question 3
What will be displayed on the page after executing the following script?
function multiplyByTwo(&$num) {
$num *= 2;
}
$a = 4;
multiplyByTwo($a);
echo $a;
A) 2
B) 4
C) 8
D) 16
Correct answer: C. This involves passing values by reference, which means the original value of variable $a will be changed.
Question 4
How do you define a constant in PHP?
A) const PI = 3.1415;
B) define(PI, 3.1415);
C) define("PI", 3.1415);
D) PI = 3.1415;
Correct answers: A, C. There are two ways to define constants in PHP.
Question 5
What value will be assigned to the $result variable?
$num1 = 10;
$num2 = 5;
$result = ($num1 > $num2) ? ">" : "<=";
echo $result;
A) >
B) <=
C) ?
Correct answer: A. This code includes a ternary operator, which is a simple way to write conditional statements.
Question 6
What type of error will you get if the file you are trying to include does not exist?
require 'file_not_exists.php';
A) Notice
B) Warning
C) Fatal error
D) Parse error
Correct answer: C. Attempting to include a non-existent file using the require statement will result in a Fatal Error.
Question 7
Which of the following mathematical functions rounds a number up?
A) floor()
B) round()
C) ceil()
D) rand()
Correct answer: C. To round a number up, you can use the ceil() function.
Question 8
How can you assign a value to a session variable in PHP?
A) create_session_variable('username', 'John');
B) $_SESSION['username'] = 'John';
C) assign_session_value('username', 'John');
D) set_session_variable('username', 'John');
Correct answer: B. This is an example of a session variable ($_SESSION).
Question 9
Which of the following statements about interfaces in PHP is true?
A) Interfaces can contain method implementations.
B) A class can implement multiple interfaces.
C) Interfaces can inherit from other interfaces.
D) Interfaces can have their own fields.
Correct answers: B, C.
Question 10
How do you send an email using PHP?
$to = "example@example.com";
$subject = "subject";
$message = "message";
$headers = "From: sender@example.com";
A) send_email($to, $subject, $message, $headers);
B) Email::send($to, $subject, $message, $headers);
C) PHPMailer::send($to, $subject, $message, $headers);
D) mail($to, $subject, $message, $headers);
Correct answer: D. To send an email, you can use the mail() function and pass all the necessary parameters.
Summary
We hope that the questions we selected have allowed you to assess your current knowledge of PHP.