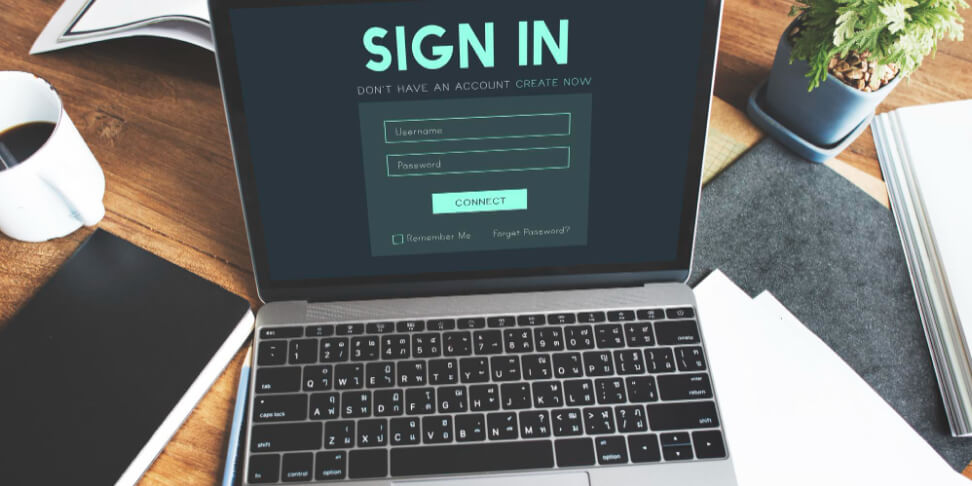
Autor: 26.05.2023
Sending forms in PHP - a basic guide
In PHP, we have two basic methods for transmitting data from forms: GET and POST. Read this article to see how to use these two methods.
GET method
We assume that you know the basics of HTML language. We have prepared a form that will send a message (the value of the name attribute in the input tag) via GET (the value of the method attribute in the form tag). The data will be sent to page.php (the value of the action attribute in the form tag).
The form looks as follows:
<html>
<head>
<title>GET</title>
<meta charset="UTF-8">
</head>
<body>
<h3>Sender</h3>
<form action="page.php" method="GET">
Message: <input type=text name="message"/><br/>
<input type=submit value="Send"/>
</form>
</body>
</html>
The above code will generate the following page.
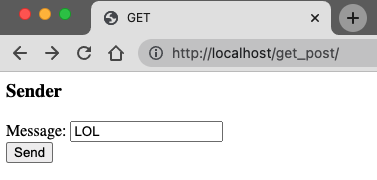
We enter the LOL message and click on the Send button. Clicking on the button will send the form. Using the GET method, we submit the data that will be visible in the URL bar of the browser.

The transfer of parameters is done in this form:
page.php[question mark][name of the parameter]=[value]
page.php?message=LOL
GET method - receiving data from the form
Remember, do not use the GET method to send passwords or sensitive data!
The data on the page.php is received using the so-called associative array. The data can be read like this:
$_GET['message']
The following example shows how to read a message:
<html>
<head>
<title>Results</title>
<meta charset="UTF-8">
</head>
<body>
<h3>Receiver</h3>
Message: <b><?php echo $_GET['message']?></b><br>
</body>
</html>
And here is the outcome of the GET request:
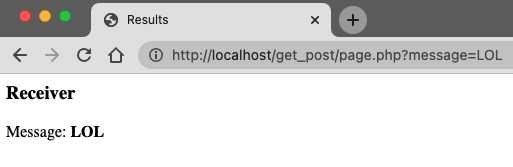
In the form, the name attribute had the name message. Using the $_GET associative array, we can retrieve this value. In this particular case, we are displaying the value stored under the index 'message'.
POST method
And now we are going to look at the POST method.
We have prepared a form that will send a message (the value of the name attribute in the input tag) via POST (the value of the method attribute in the form tag). The data will be sent to page.php (the value of the action attribute in the form tag).
The form looks as follows:
<html>
<head>
<title>GET</title>
<meta charset="UTF-8">
</head>
<body>
<h3>Sender</h3>
<form action="page.php" method="POST">
Message: <input type=text name="message"/><br/>
<input type=submit value="Send"/>
</form>
</body>
</html>
The above code will generate the following page.
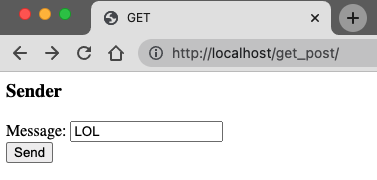
POST method - receiving data from the form
We enter the LOL message and click on the Send button. Clicking the button will send the form. Using the POST method, we submit the data using the request header. This time we will no longer see the data in the URL of the web browser.

The transmission of parameters is done this way. This is a screenshot from the browser console:
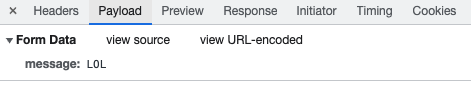
We retrieve the data on the page.php using an associative array. We read the data using the array like this:
$_POST['message']
The following example shows how to read a message:
<html>
<head>
<title>Results</title>
<meta charset="UTF-8">
</head>
<body>
<h3>Receiver</h3>
Message: <b><?php echo $_POST['message']?></b><br>
</body>
</html>
And here is the result of sending a POST request:
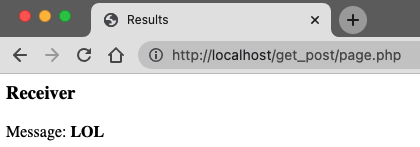
The name attribute has the value of message. Using the $_POST associative array, we can read submitted parameters from the form. In this particular case, we are displaying the value stored under the 'message' index.
Summary
We have two basic methods for transmitting data from forms: GET and POST. Receiving data shouldn't be a major issue; it's a matter of using the appropriate variable. Of course, in practice, there are very important issues related to security and validating the correctness of this data. But that's already a topic for a separate discussion.
If you want to learn PHP from scratch and acquire the skills needed for a Junior PHP Developer position, start your learning journey with the Career Path. The learning concludes with an exam that qualifies you to use the Certificate of Completion.