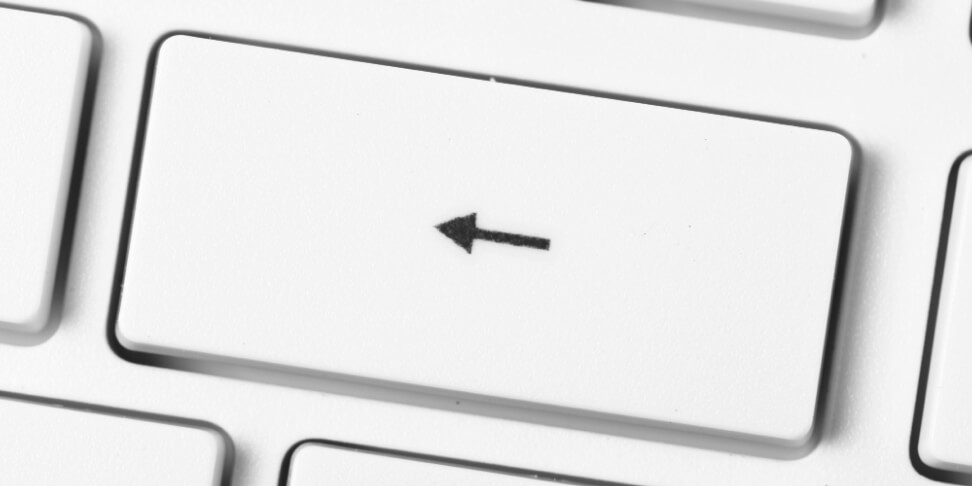
Autor: 22.03.2023
The essentials of pointers
The topic of pointers is a recurring one amidst C and C++ programmers, as it is a pretty important element in those languages. At the same time, pointers prove to be a pretty mysterious or even abstract thing for beginners. In this article we’re hoping to resolve some of your doubts around pointers, and help you better understand the big idea behind using them in programming.
Let’s define pointers
A pointer is a variable that points to another variable’s address, hence the name - pointer. Your traditional variable stores values like:
- A variable named x could store a value like 1
- A variable named score could store a value like 200
- A variable named user could store a value like “Admin”
Pointers, on the other hand, store the variables’ addresses. What’s the address then? Let us tell you.
The array of addresses
With some programming languages you could think of the computer’s memory as an array. The array stores all the data from our program. As you may know already, in programming arrays have indexes. You could imagine a pointer as such index, that allows you to access a specific element of the array.
So to summarize: we have an array of variables, and each element of the array has its own index (its own address), that we can access with pointers.
How it works - a simple example
Now let's take a look at a C++ example with pointers. We chose this specific language because it’s popular and will also allow us to easily visualize our main concepts. Here’s the code:
int *p;
We’ve declared a pointer named p, that points to values of type int. So - what do we do with a pointer like that? Take a look at the complete code:
int *p;
int digit = 7;
p = &digit;
std::cout << p;
We’ve added another variable named digit, that stores the value 7. Now, the next line is key: p = &digit. And this is what this notation means: pointer p is pointing to the address of the digit variable. Not the value, the address - that’s very important! Now, what happens when we run the code?
0x505278
This weird looking string of characters is the address of the digit variable in the computer’s memory. You could say that the digit variable is stored under the address of 0x505278. We learned this thanks to our p pointer - this is exactly what pointers are for. But what do we do with this knowledge? Let's talk about this.
Examples of usage
Pointers have their purpose - especially when the more standard features of the language won't cut it. It’s worth noting that the purposes greatly depend on the language. For example, C++ is a higher-level language than C, so the need for using pointers arises less frequently. In C on the other hand, pointers fulfill a much more important role - they allow us to bypass the limitations of the language’s features.
There are some areas where pointers can be really helpful.
- Code efficiency optimization - pointers, when used correctly, can be more efficient than the alternative solutions.
- Low-level programming, e.g. in nested systems. Here pointers could sometimes be the only way to reach the set goal.
In C, pointers are often used for:
- returning more than one value from a function,
- processing dynamic arrays, if we do not know their size;
These are only a few examples that are supposed to show the usual usages of pointers.
Important note though. Pointers in general require a responsible approach. Their very nature makes them susceptible to causing many, hard to diagnose errors. It can be a blessing when you have access to a variable’s address, but it can also make it easier for us to accidentally overwrite a value when we least expect. Simply put - it’s a tool that demands a careful and reasonable approach.
What languages use pointers
Typically, pointers find their use in C and, a little less so, in C++. They also appear in other low-level languages, e.g. Assembler, and play a very important role in them too.
The more high-level the language, the less of a role pointers have to plan. There are some languages that use pointers in an implicit way too. They’re used by the inner mechanics of a language, and the programmer cannot access them.
Summary
Pointers are a special kind of variable that point towards the addresses of other variables. In some languages they play a big part, like they do in C, while in others the pointer mechanics don't even exist.
Pointers are a tool that needs to be used responsibly. Sadly, they do not grant you magical abilities and make the code more efficient from the get-go, and when used incorrectly, may cause more harm than gain. Of course they’re very much worth getting the hang of, and especially so if you plan on working in an environment that allows you to utilize their advantages.