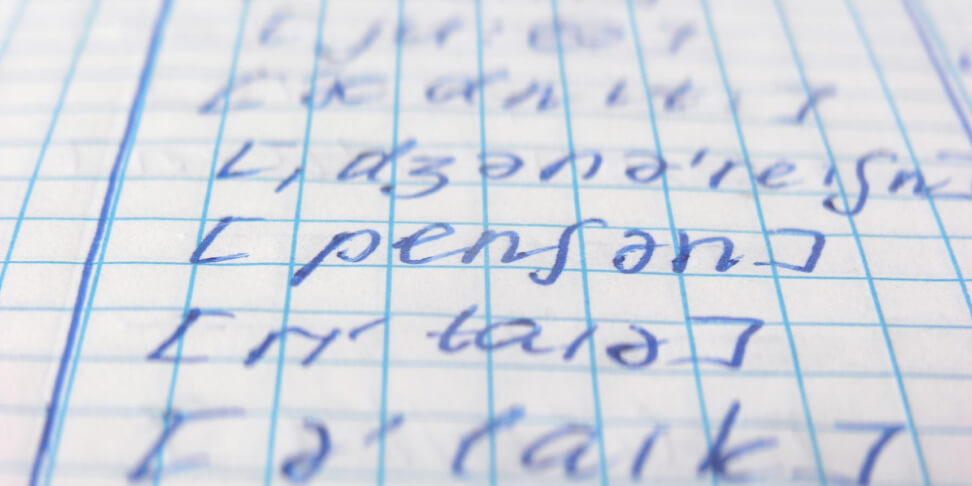
Autor: 25.04.2023
The naming conventions of C#
Many programming languages have their own naming conventions. They set the guidelines for how we name objects, classes, variables etc. Thanks to that, we are able to achieve a level of code consistency across our programs. When code is written according to the set conventions, it’s clearer, more readable and makes teamwork easier.
In this article we will go over the main naming conventions in C#.
Pascal case
The notation known as Pascal Case is used, among other things, for naming classes, methods and properties. All words in the name should start with an uppercase letter. Here’s an example:
ClassName {
}
Camel case
This notation is only slightly different from the previous one, the difference being: the first word starts with a lowercase letter. In C# it’s mainly used when naming private fields:
private int _speedMax;
Sometimes private fields also get an underscore (“_”) at the beginning of the name, as visible in the example above.
Uppercase letters
Names consisting of uppercase letters only, are what we use for constants. Take a look:
public const double PI = 3.141592653589793238;
That’s all on the notation essentials! Now we’ll go over which one to use in what situation.
Key rules
Take a look at the examples below. They encompass most of the C# typical elements.
- Classes, interfaces and structures: use PascalCase.
- For interfaces, adding an “I” prefix is advised, e.g. ICollectable.
- Methods: use PascalCase, e.g. GetCarInfo().
- Properties: use PascalCase.
- Events: use PascalCase.
- Private fields: use camelCase. Sometimes a “_” prefix is added, e.g. _privateField.
- Public fields: use PascalCase.
- Parameters: use camelCase.
- Constants: use UPPERCASE LETTERS with an underscore (_).
- Local variables: use camelCase.
As you can see - three basic conventions: PascalCase, camelCase and UPPERCASE LETTERS. Everything else is just making sure to fit the right convention to the elements in the code :)
Example - the Employee class
Take a look at the example class. It doesn't contain all of the before mentioned elements, but the most important ones are present.
public class Employee
{
// Private field
private decimal _monthlySalary;
// Public field
public string FirstName { get; set; }
// Public field
public string LastName { get; set; }
// Public field readonly
public decimal MonthlySalary
{
get { return _monthlySalary; }
}
// Constructor
public Employee(string firstName, string lastName, decimal monthlySalary)
{
FirstName = firstName;
LastName = lastName;
_monthlySalary = monthlySalary;
}
// Method calculating sallary
public decimal CalculateAnnualSalary()
{
return _monthlySalary * 12;
}
// Method applying raise
public void ApplyRaise(decimal raisePercentage)
{
if (raisePercentage > 0)
{
_monthlySalary += _monthlySalary * (raisePercentage / 100);
}
}
// Method returning info about employee
public string GetEmployeeInfo()
{
return $"First Name: {FirstName}, Last Name: {LastName}, Monthly Salary: {MonthlySalary}";
}
}
Take particular note of the key elements. The class has fields storing employee information: FirstName, LastName and _monthlySalary. It also has some methods, like CalculateAnnualSalary(). All were named according to the widely known and accepted naming conventions for C#.
Of course, you still need to remember about the universal rules in programming that are not language-dependent. Things like readability or using unambiguous names that describe the code elements accurately.