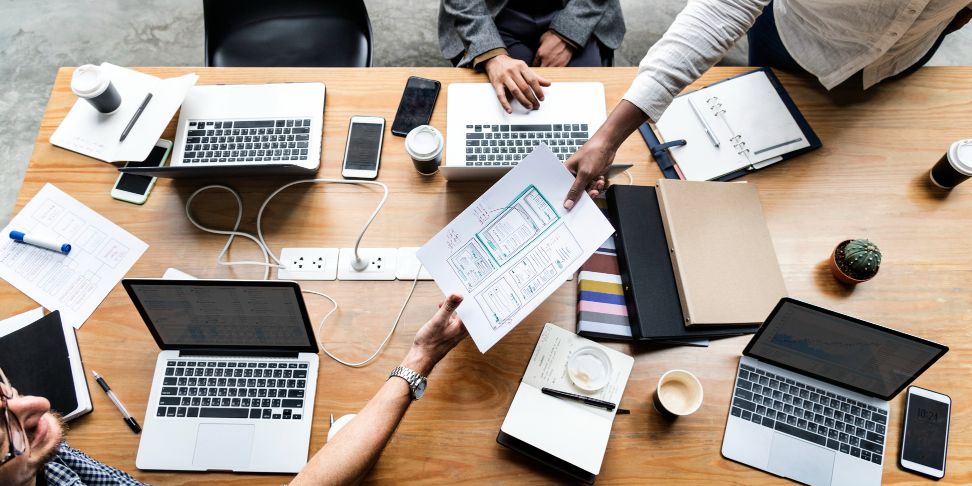
Article by: 26.07.2022
What is structured programming
Structured programming is a programming paradigm that puts emphasis on a linear, easy to understand structure of the source code. The order of execution should be the same as the order of the source code.
How does it work?
Let’s take a closer look at this “linear flow” concept. Imagine, that you are analyzing a source code of some program. If the source code is written with a good structure then you will be able to quickly build a mental model of the execution of this code.
You will be able to easily find the entry point of the program. You will be able to easily find the exit point of the program. You will be able to trace the execution of the program line by line.
The way the code is executed will reflect the way the source code is ordered. There won’t be any sudden ‘jumps’ in the code. Get the idea?
Simply imagine that you can easily trace the way the code is run just looking at its source code.
Main elements of structured programming
While looking at the source code created with structured programming principles in mind, we can find some recurring elements. These elements can be broken down into a few main categories. So let’s take a look at them now.
Blocks
A block is a set of related statements or instructions. Think of any part of the code that performs a single task. It can be a function taking some arguments and returning a result. Or it can be a loop iterating over the list of values. Just anything.
A proper block should have a single entry point and a single exit point. That's a very important feature. You can imagine that we “enter” the block, run some instructions and then we exit the block.
This approach greatly simplifies debugging and testing our code. When we give a correct entry data and we get an incorrect result, then we can be sure that there is an error inside of the block.
Repetition (iteration)
Repetition is usually implemented using loops. Let’s stop for a moment and think: can you build a properly structured program with loops? Sure - you can!
Try to think of the loop as the block of code. We enter the loop, iterate over some values and then we exit the loop. There is nothing complex here. Everything is built on a simple, linear flow.
It doesn’t matter how many times our loop will run. It may run dozens of times, it may run thousands of times. As long as there is a single entry point and a single exit point, we can say that the loop fulfills the criteria of structured programming.
Selection
Selection is usually implemented with some kind of an if statement. You may already be familiar with a very common IF-ELSE statement. That’s a good example of a selection mechanism.
What about structured programming? Can you use IF statements while writing a “structured” program?
We usually start the if statement with a condition - that’s the entry point. If the condition is true we run a block of code and exit it. If the condition is false we run a different block of code and exit it.
So we got blocks, entry points, exit points etc.These are standard elements of structured programming.
Nesting
You can easily nest blocks of code. Smaller blocks can be nested inside larger blocks. These nested blocks should have a single entry point and a single exit point. There is really nothing surprising about this. You can create complex structures based on multiple levels of nesting. As long as you build your code with structured programming principles in mind you are good to go.
What is an antithesis of structured programming?
Now we will talk about the infamous Go To statement. Nowadays it is rarely used and for a good reason. The Go To statement is simply the antithesis of structured programming.
This statement can transfer the control from one line of code to another. When the code is executed, the control of the flow can suddenly jump from one place to another. It can jump eg. from line number 12 to line number 340. It can jump from line number 34 to line number 321. Get the idea?
Such code is pretty hard to follow and equally difficult to debug. It breaks the linear flow. That’s why the Go To statement has fallen out of favor many, many years ago. And that’s why the whole paradigm of structured programming was created.
Structured programming in practice
There are only a few languages that force you to program in a structured style. Majority of programming languages will allow you to freely use all common programming styles and paradigms. If you want to stick to the structured programming rules then it's your responsibility to implement them properly.
Structured programming principles can be implemented in almost any programming language. You can use it in Java, Python, C++, JavaScript etc. As we said before, the language itself has nothing to do with structured programming. It’s up to you to properly design and implement your code in a structured way.
It’s a very deep topic. You can implement structured programming rules using different approaches: OOP, functional programming etc. Remember that it all boils down to some basic rules that we have discussed in this article.