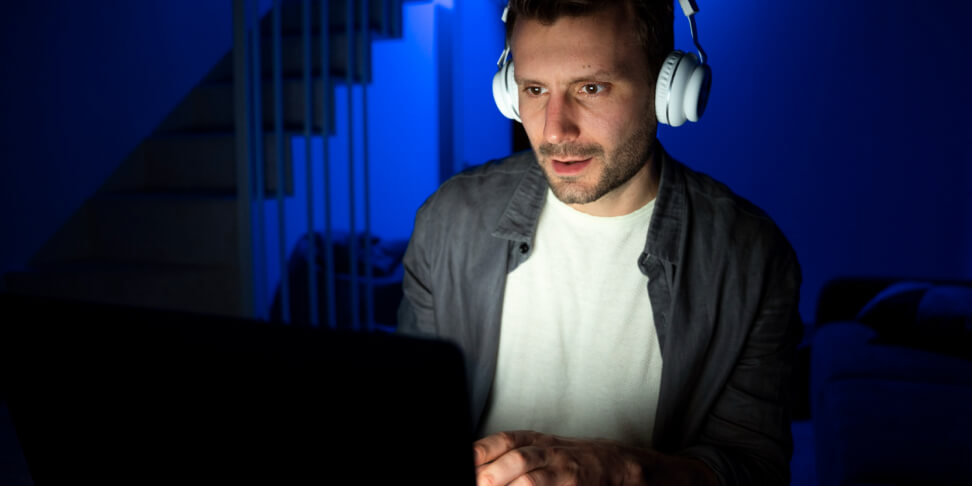
Autor: 16.01.2024
C Language - File Operations
The C language provides built-in functions for performing basic file operations. These operations include opening a file, writing data to it, reading its contents, etc. In this article, we will show you how to perform these basic operations.
Standard File Operations
Before we begin, it's important to mention a crucial thing. We typically use file operations in the following order: first, we open the file, perform operations (read or write), and finally, we close the file. Remember this order.
Opening and Closing a File
Let's start with the most important operation, which is opening a file. We need to open it to work with it. Here is what the code looks like:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("my_file.txt", "r");
fclose(file);
return 0;
}
Pay attention to the key elements:
- FILE *file; is a file pointer. We simply named it 'file.'
- file = fopen("my_file.txt", "r"); calls the file-reading function. We have the parameter "r," which means we are opening the file for reading. Our file is named my_file.txt.
- fclose(file); closes our file. It's always good practice to close the file after finishing all operations to free up memory resources.
At the moment, our code doesn't do much. It simply opens and shortly after closes the file.
Writing to a File
Now let's try to write data to our file. Take a look at the modified code:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("my_file.txt", "r");
fclose(file);
return 0;
}
Notice the parameter of the fopen() function. This time it's "w" ("write") - we need to open the file in a mode that allows writing. The new element in the code is the fprintf() function, which is used to write text to the file. Finally, we add a newline character \n. After completing the write operation, the file will be closed using fclose().
Reading a File
Now that you know how to write data to a file, let's try to read it. Here is our new code:
#include <stdio.h>
int main() {
FILE *file;
file = fopen("my_file.txt", "r");
char buffer[100];
while (fgets(buffer, sizeof(buffer), file) != NULL) {
printf("%s", buffer);
}
fclose(file);
return 0;
}
And here is the result returned by our code:
This is text that will be written to the file.
There's nothing surprising here - the text written to the file in the previous example has been read. Now, let's analyze the new read operations:
- We open the file in reading mode, i.e., "r."
- We create a character array named buffer to store the read content of the file. Its size is 100.
- Using a while loop, we read the file line by line.
- The fgets() function reads a line from the file (file) and puts the read data into the buffer array.
- We have a loop termination condition: != NULL. The loop runs as long as there are lines to read (until NULL appears).
- We print the read line to the screen using the printf("%s", buffer) function.
Of course, the file is closed at the end - as you already know.