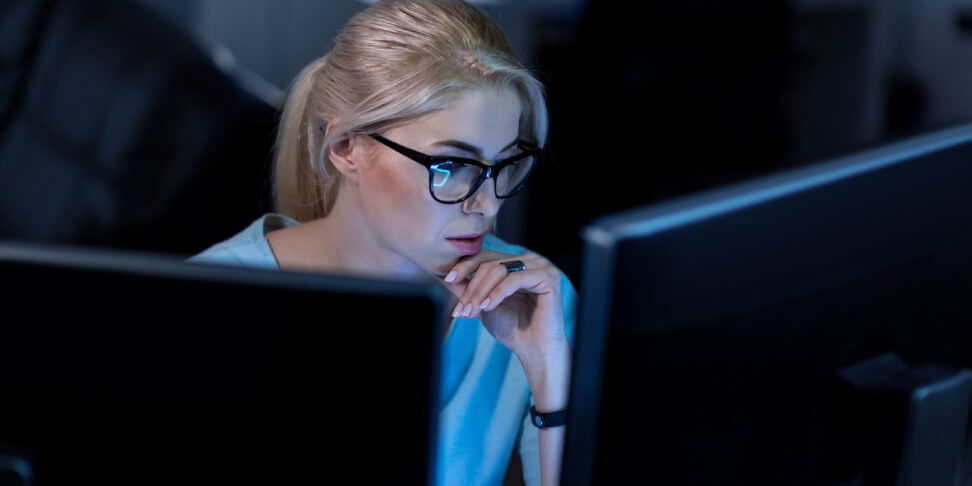
Autor: 20.12.2023
C# - Abstraction and Encapsulation
The C# language is inherently object-oriented. In object-oriented programming, there are certain rules that enable us to create more readable and easier-to-maintain code. In this article, we'll present two such rules: abstraction and encapsulation. We'll show examples of code created using these two important concepts.
What is Abstraction and How to Understand It
Abstraction is a concept involving hiding complex details and showing only the essential functions of an object. It's akin to using a car: you don't need to precisely understand how the engine works to drive a car. Knowing how to use the steering wheel, pedals, and other basic control elements suffices.
A Simple Example of Abstraction
Let's imagine creating a program to manage vehicles. We start by creating an abstract base class called Vehicle, which contains basic properties and methods common to all types of vehicles.
public abstract class Vehicle
{
public abstract void StartEngine();
public void StopEngine()
{
Console.WriteLine("Engine stop");
}
}
The Vehicle class contains an abstract method StartEngine, which must be implemented in derived classes, and a regular method StopEngine, which already has its implementation.
Next, we create a concrete class Car that inherits from Vehicle and implements the StartEngine method.
public class Car : Vehicle
{
public override void StartEngine()
{
Console.WriteLine("Engine start");
}
}
How to use such an abstraction? Here's an example:
class Program
{
static void Main()
{
Vehicle myCar = new Car();
myCar.StartEngine();
myCar.StopEngine();
}
}
In the above example, Car is a specific vehicle that implements the abstract behaviors defined in Vehicle.
Summary
Abstraction is a way to hide complex details and show only what's necessary. An abstract class in C# can contain abstract methods (without implementation) and regular methods. Derived classes implement abstract methods and can use regular methods from the abstract class. This makes your code more organized and easier to manage.
What is Encapsulation
Encapsulation in C# is a way to hide the implementation details of a class and expose only what's needed for its usage. It can be compared to a TV remote: as a user, you know functions like power on/off, channel change, or volume adjustment, but you don't need to know how the TV works inside. Encapsulation allows hiding the "inside" of an object and exposing only elements safe to use.
A Simple Example of Encapsulation
Let's say you have a BankAccount class. You want the account balance to be secure and changed only in a controlled way.
public class BankAccount
{
private decimal balance;
public void Deposit(decimal amount)
{
if (amount > 0)
{
balance += amount;
}
}
public bool Withdraw(decimal amount)
{
if (balance >= amount && amount > 0)
{
balance -= amount;
return true;
}
return false;
}
public decimal GetBalance()
{
return balance;
}
}
What happens in the above code?
The private field balance is a private field, meaning its value can only be accessed within the BankAccount class. This prevents accidental or unauthorized changes to the balance. The Deposit(), Withdraw(), and GetBalance() methods are public methods that allow interaction with the bank account. You can deposit and withdraw money and check the balance, but you can't directly change balance. Access Control: The BankAccount class user can use public methods but doesn't have direct access to the internal state (account balance), which is the essence of encapsulation.
Summary
Encapsulation is like having a safe in a bank. You can put in or take out money (Deposit() and Withdraw() methods) and check how much you have (GetBalance() method) but don't have direct access to the internal mechanisms of the safe that protect your money.
Final Thoughts
We hope that after reading this article, you understand what abstraction and encapsulation entail. These are widely used object-oriented programming techniques. They enable the creation of better, safer, and more predictable code.