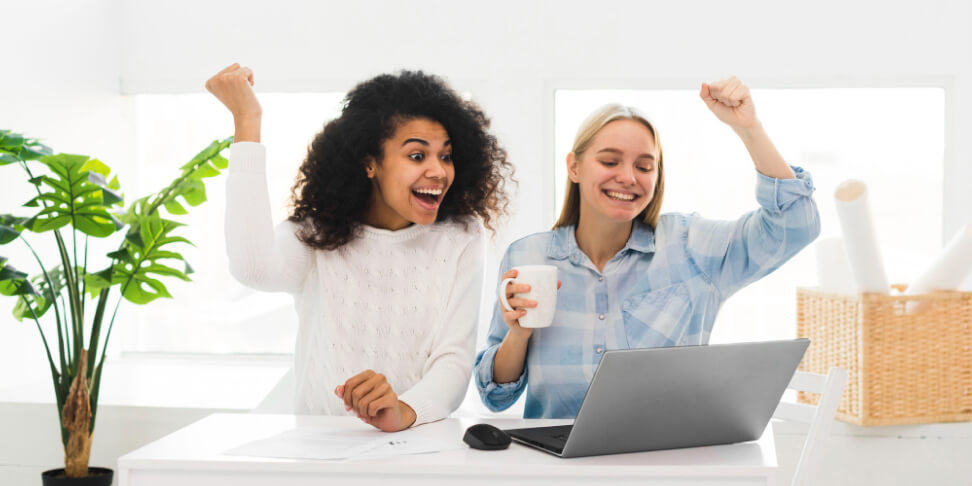
Autor: 19.02.2024
C# - Four Coding Challenges For Beginners
Here are four C# coding challenges for you to practice. They are a great way to hone your coding skills. Each challenges starts with a task description and excepeted results. Your task is to implement a C# functions that will return expected results. The challenges are meant for beginners. If you have mastered the basic C# concepts like functions, loops, artithmetical operations, you should have no problems dealing with those tasks. Practice your skills to become a Junior C# Developer. Good luck!
1. Sum the odd numbers
Implement an algorithm to sum together all the odd numbers in a collection. Here are the tests that your solution should pass:
- new App().SumOdds(new int[]{1, 2, 3, 4, 5}) -> 9
- new App().SumOdds(new int[]{1, 2, 3}) -> 4
- new App().SumOdds(new int[]{2, 2}) -> 0
- new App().SumOdds(new int[]{1, 1}) -> 2
That one should be fairly easy. You just need to check each number in the collection, to see whether it is odd or even. If it is odd, then add its value to the sum.
Here is the initial code to give you a headstart:
using System;
public class App {
int SumOdds(int[] digits) {
int sum = 0;
// your code here
return sum;
}
static void Main(string[] args) {
}
}
2. Factorial
Here is the classic programming challenge. What is a factorial?
A factorial is a mathematical operation that is applied to a non-negative integer. The factorial of a non-negative integer "n," denoted as "n!," is the product of all positive integers from 1 to "n." In other words:
n! = n × (n - 1) × (n - 2) × ... × 2 × 1
For example:
5! = 5 × 4 × 3 × 2 × 1 = 120
0! is defined to be 1
Take a look at the tests of the Factorial() function. The expected results:
- new App().Factorial(0) -> 1
- new App().Factorial(1) -> 1
- new App().Factorial(2) -> 2
- new App().Factorial(3) -> 6
And here is the initial code. you just need to finish the implementation of the Factorial() function:
using System;
public class App {
int Factorial(int digit) {
// your code here
}
static void Main(string[] args) {
}
}
3. Convert hours to days
Your job for now: convert hours into days. Here is the basic formula: days = h / 24.
Here are the tests that your function should pass:
- new App().ToDays(24) -> 1
- new App().ToDays(6) -> 0,25
- new App().ToDays(12) -> 0,5
- new App().ToDays(48) -> 2
And, as always, we are giving you an initial code:
using System;
public class App {
double ToDays(double hours) {
// your code here
}
static void Main(string[] args) {
}
}
That one should be very easy!
4. Count words in text string
And the last one - counting words in a given text string. Let’s start with tests:
- new App().CountWords(null) -> 0
- new App().CountWords("Hello") -> 1
- new App().CountWords("Hello Codenga") -> 2
- new App().CountWords("Hello Codenga !!!") -> 3
And the initial code:
using System;
public class App {
int CountWords(string message) {
// your code here
}
static void Main(string[] args) {
}
}
Some hints: split the string (message) into separate words, count the number of words, return the result. And that’s it!