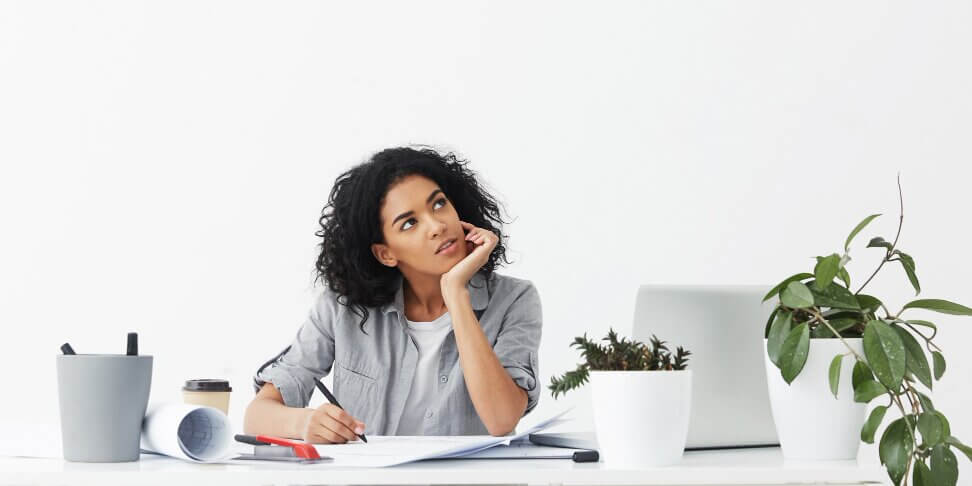
Autor: 10.11.2022
C# - Introduction to LINQ queries
This article is a comprehensive introduction to the topic of LINQ queries. LINQ can make working with data a whole lot easier. It’s a very important part of C# ecosystem and the NET platform. Thanks to LINQ we can transform all data in the same, universal way, no matter what their source is.
The sources for data in applications can be varied - it could be an XML file or a SQL database. Alternatively, it could simply be other parts of our app that themselves communicate with an external server.
With LINQ you don't have to worry about where the data comes from. You just process it the same way - regardless of where it came from.
If you work with NET, it’s crucial to know how to use LINQ queries. Let’s begin!
Query syntax and method syntax
LINQ offers two basic syntaxes: the query syntax and the method syntax. Treat them as two means to reach the same goal.
In practice you will most likely be using just one or the other. Ultimately it comes down to which one you prefer, but from time to time you might stumble upon a project that enforces a specific syntax. In that case, it’s good to be familiar with both of them.
How to start with LINQ
To start working with LINQ, you need to use the right namespace:
using System;
using System.Linq;
These are the standard declarations that need to be added at the beginning of our code. They allow us to freely use everything LINQ has to offer.
WHERE queries
Let's get into an example straight away, here’s the code we’re working with:
using System;
using System.Linq;
public class Program
{
public static void Main() {
var numbers = new int[5] { 7, -4, 2, -1, 0 };
var numQuery = numbers.Where(n => n > 0);
foreach (var num in numQuery)
{
Console.WriteLine(num);
}
}
}
Here we have a simple collection of int values - this is our data source. Now, we create a new query:
var numQuery = numbers.Where(n => n > 0);
Take notice of the Where() operator - it allows us to only choose the values that fulfill a specific condition. In this case we want our data to be positive numbers, so the below lambda expression is passed to the operator:
n => n > 0
The n element of course stands for one single value. At this stage, the numQuery variable contains a filtered collection of values (just the ones higher than 0). The only thing left to do is to return the result to the console using a loop. Here’s the final result:
7
2
We get two values that fit our criterion.
The main advantage of LINQ is how simple and compact it is. Using one simple operator we were able to filter data out of a collection - and this is just the beginning of what LINQ has to offer.
TAKE queries
Let’s go over another example:
using System;
using System.Linq;
public class Program
{
public static void Main()
{
int[] digits = { 1, 2, 3, 4 };
var query = digits.Take(2);
foreach (var q in query)
{
Console.WriteLine(q);
}
}
}
This time we used the Take() operator. It allows us to get a specified number of elements from the beginning of our collection. We passed 2 as the parameter, and so we get the first two values:
var query = digits.Take(2);
It’s easy to predict the result:
1
2
Take() is a simple, yet an extremely useful operator. It showcases the entire philosophy of LINQ - with simple operators we can process data collections to our very hearts content. For clarity’s sake, in this article we chose to focus on some non-complex examples. In practice though, you can chain those basic operators into any and all combinations.
Syntax once again
In the examples above we have been using the so-called method syntax. As we’ve mentioned, there is another one - the query syntax. Take a look at an example:
var numQuery =
from n in numbers
where n > 0
select n;
Here we have a snippet that, you might notice, is pretty reminiscent of a SQL query. What’s interesting though is that the result of this query is going to be exactly the same as the one from our first example - we’ll get the values greater than 0. As you can see, it’s just a different way to reach the same destination.
Summary
LINQ is a simple tool with great capabilities. Using simple operators you can perform any operations on data, and it doesn’t matter where the data comes from.
LINQ is an important part of the .NET platform. If you intend to go deeper into this technology, getting to know LINQ better will be an essential step towards success.