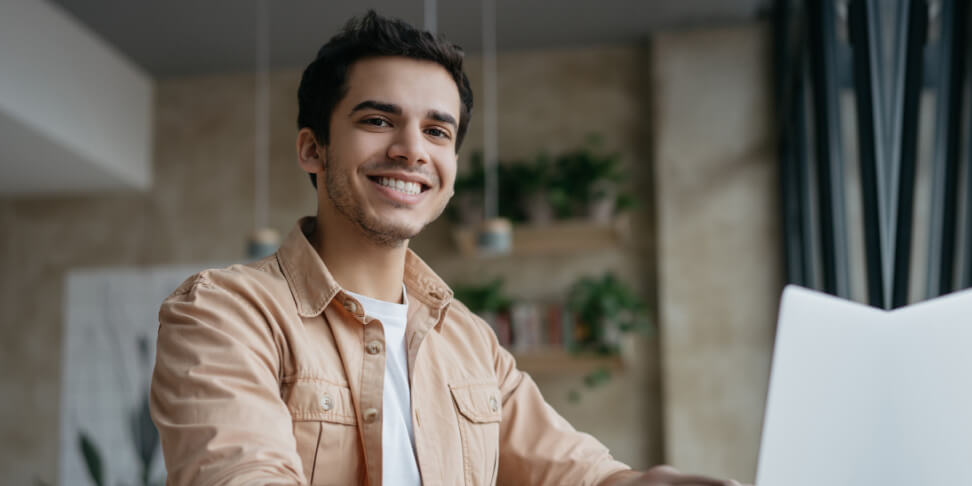
Autor: 24.11.2022
Functional programming - what’s it all about?
There’s been a lot of talk lately about functional programming. So - what’s the big idea behind the functional approach?
The main component making a program up, in functional programming, are functions. But there’s a catch - they need to fulfill a couple of key conditions first.
First and foremost, each function should only be able to do one thing. Long story very short, there’s a simplistic way to describe it: input data → data gets processed → output data.
One function doing one thing won’t get us very far though, and this is where the main idea shines through - we need to make a composition. That means simply taking the data returned by one function and feeding it to another, easy :)
This approach to writing code surely has its perks - because we are using simple functions, performing singular operations, the program is easy to test. Every function we can easily isolate and see if it works as expected.
Smaller pieces also mean it’s simple to put them together into bigger blocks of code. That, in turn, allows us to reuse what we already have multiple times. Hurray time economy!
You might have come across the information that the functional approach allows writing code declaratively. What this means, in short, is that you can focus more on what goal you want to reach instead of the steps needed to get there - you laser in on the destination instead of the road leading up to it. By using simple functions to build code, we clearly “declare” what we want to do. There are also benefits in the form of a clearer and more readable code.
Functional programming across languages
In practice the functional approach can be used in many different programming languages. Most of the popular languages allow for various approaches in coding: object-oriented, functional etc, you take your pick.
There are also languages that, in a way, enforce a certain approach, e.g. Haskell. Keep in mind most of the time those are the niche languages, functional approach is very much viable in every big language: Java, JavaScript, PHP, C# etc.
The mechanics of functional programming
There are a few rather specific mechanics that functional programming is based upon, and you need to learn them if you want to use the functional approach in your code. Let’s take a look at a few examples.
Pure functions
We’ve mentioned already that functional programming heavily relies on functions that each perform one job. These are often called “pure functions”, and have their characteristics.
- The returned result only depends on the input data.
- For the same input data, the function will always return the exact same result.
- A pure function doesn’t have any “side effects”. It doesn’t modify global values and its result is not dependent on external factors that we are not in full control of.
Here’s a simple example:
function add(a, b) {
return a + b;
}
The function that takes two parameters and returns their sum. It does not modify any variables that exist outside of its scope and its result is only dictated by the input parameters. That is what a pure function is.
Composition
We’ve talked about compositions a bit already. The main idea is: you take simple, pure functions and piece them together into bigger blocks. By doing that you simply put values through a chain of functions, each taking you one step closer to the desired result.
Other techniques
Functional programming as a topic is a very vast one. There are many mechanics that would need their own articles to fully explore what they entail - ones known as partial application or currying.
Example - mapping
The technique known as map is a great example of functional programming mechanics. Let’s say we have a list of values and we want to square them. Take a look at our example:
let digits = [ 1, 2, 4, 5, 8 ];
let squares = digits.map(digit => digit * digit);
To achieve our goal we put to use some features of JavaScript. The map() function allows us to execute an operation on each element of a list or array. The operation we’re interested in this time around is squaring, so digit => digit * digit. The result we get is a processed list of our values:
[ 1, 4, 16, 25, 64 ]
Notice that we have achieved a simple and readable code - it’s very declarative. We declared what we want to achieve, in our case it’s values squared. At the same time we also avoided writing complex loops and other, hard to analyze mechanics.
Practical uses
Before we go, let’s talk about some real usages of functional programming.
This approach is simply an alternative to all the other ones. Nearly every application can be written in a functional way - though it’s not always the most sensible way to go about it. In the past few years, functional programming has seen a distinct increase in popularity. It’s gone as far as to step over into languages that have always been rooted in other approaches.
If you work with JavaScript, functional programming is something you’ll probably be running into quite often. Countless applications written in that language are based on - you guessed it - the functional approach. The React framework is one of the many examples, as there’s more and more of them with every day, and especially throughout the few recent years.