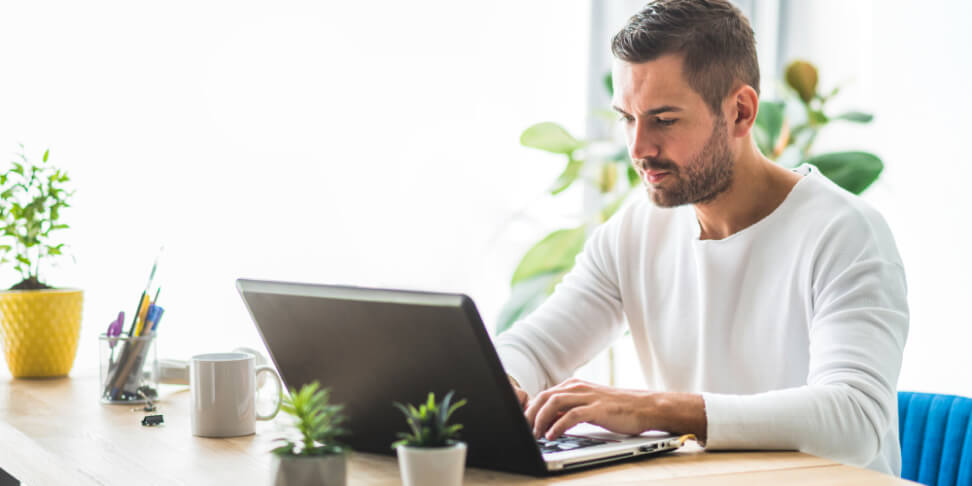
Autor: 29.06.2023
Golang (Go) - simple tutorial for beginners
Go (also known as Golang) is an open-source programming language developed by a team of engineers at Google. Go is characterized by its simple syntax, designed to be readable and understandable. This language has gained popularity among programmers due to its simplicity, performance, and support for concurrency.
In this article, we will show you how to write your first program in Go. We will be writing a program that calculates the arithmetic mean of a given list of numbers.
Tools you will need
To run the code from this article, you can simply use a straightforward online compiler available at this address: https://go.dev/play/. This way, you can avoid the need to install and configure a local development environment.
Go - key concepts
At the beginning, we will explain the most important concepts of the language that will be necessary to write our simple program.
Go packages
In Go, programs are organized into packages, which contain a set of related source files. Each source file in a package should have the same package name declared at the beginning.
Packages in Go are declared as follows:
package main
Such a declaration indicates that the source file is part of the "main" package and contains the "main()" function, which serves as the entry point of the program.
You can import other packages that are needed in your program. For example:
import (
"fmt"
)
We import the "fmt" package, which is commonly used in Go for text formatting and input/output operations. With this package, you can, among other things, print data to the console.
A main() function
Every Go program has a special function called "main()" which serves as the entry point. The program execution starts from this function. It is automatically executed when the program is run. We declare the "main()" function as follows:
func main() {
// function code goes here
}
Variables
Variables are used to store values. Here is an example:
sum := 0.0
We have created a variable named "sum" with an initial value of 0.0. It is worth noting the use of the := operator (colon and equal sign). This is a distinctive element of the Go language. It allows us to declare a variable and assign a value to it simultaneously.
Slices
In Go, we have another interesting element called a "slice." It allows us to store a dynamic list of multiple values. You can think of a slice as a variable that can hold more than one value. Take a look at the code:
numbers := []float64{4.5, 2.3, 6.7, 8.1, 5.2}
We have created a list of numbers and assigned it to the variable "numbers". The numbers in this list are of type float64, which indicates they are floating-point numbers. We have provided the numbers using curly braces {}.
Go functions
Functions are blocks of code that can be reused multiple times. In our program, we will calculate the arithmetic mean. Therefore, we will create a function that performs this operation.
func calculateAverage(numbers []float64) float64 {
}
Our function is named "calculateAverage." It takes one parameter named "numbers," which should be a slice of floating-point numbers. The function returns a floating-point value of type float64.
Loops
Using a loop, we can repeat a specific action. In the case of our program, we will use a loop to sum up the list of numbers. This way, we can later divide the sum by the number of elements to obtain the average.
Here's an example of a loop:
for _, num := range numbers {
sum += num
}
Yes, it is a "for" loop that processes the "numbers" list. Each element from the list is represented by the variable "num." With each iteration, we add the current number to the "sum" variable using the expression "sum += num." The loop continues until all the numbers in the list have been processed, at which point the loop terminates.
Final code
At this stage, you are already familiar with the main programming concepts in the Go language, at least those that will be needed to write a program that calculates the average. Now, let's take a look at the final code of the program:
package main
import (
"fmt"
)
func main() {
numbers := []float64{4.5, 2.3, 6.7, 8.1, 5.2}
average := calculateAverage(numbers)
fmt.Println("Average:", average)
}
func calculateAverage(numbers []float64) float64 {
sum := 0.0
for _, num := range numbers {
sum += num
}
average := sum / float64(len(numbers))
return average
}
Can you paste the code into the online editor mentioned at the beginning of the article? After clicking the "Run" button, the program will be compiled and executed. The following result should appear: Average: 5.36.
Let's take a closer look at the main elements of the program once again.
- We declare a package named "main" and import the "fmt" package.
- Inside the main function, we create a slice (list) of float64 numbers and assign some sample numbers to it.
- We create a variable named "average" to store the calculated average. We assign it the result returned by the calculateAverage() function.
- Using fmt.Println("Average:", average), we print a message and the value of the "average" variable to the screen.
Now let's take a look at the operations inside the calculateAverage() function:
- We declare a variable named "sum" to store the sum of all the numbers.
- Using a "for" loop, we sum up all the numbers.
- We assign the average to the variable "average" by dividing the sum by the number of elements. We determine the number of elements using the built-in "len()" function, which returns the length of the "numbers" slice.
- At the end of the function, using the "return" statement, we return the value of the "average" variable.
Summary and next steps
This simple program should allow you to take your first steps in the world of Go. Feel free to experiment with it—for example, you can change the list of numbers to different values and see what the result will be.
If you want to learn the basics of Go in an effective and practical way, I recommend starting with the Fundamentals of Go - Level 1 course. It offers a lot of exercises, tasks, and accessible theory to help you get started.