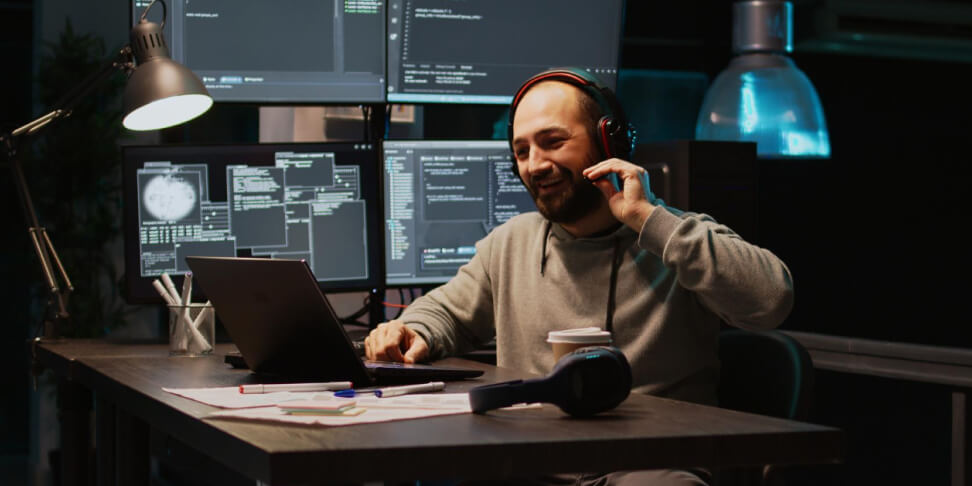
Autor: 23.02.2024
How to Write Good Code Comments
Writing good code is constantly talked about. Countless programmers are always discussing design patterns, variable naming conventions, and so on. However, not much time is dedicated to writing good comments in code. Yet, this is a very important part of our work. In this article, we will discuss several simple and important rules for writing good comments.
Good code is code that is understandable for people
Poorly written comments can be worse than no comments at all. So, how do you create good code comments?
Comments should not duplicate code
Beginner programmers are often taught to comment on as much code as possible, which can lead to an overabundance of comments explaining every possible fragment of code, even if it's very simple and understandable to everyone.
score = 10 # assigning to variable a
This example illustrates the phenomenon of excessive commenting. Why explain with a comment something as obvious as assigning to a variable? Such a comment adds nothing and the effort put into creating it is wasted. Consider carefully whether the comments you write actually contribute something meaningful.
Comments should not replace good code
Let's return to the variable example:
x = 1 # initial counter state
The variable x is poorly named. If it were named correctly from the start, the comment wouldn't be necessary. Since it represents the initial counter state, let's name our variable accordingly:
initial_counter = 1
Now the comment is unnecessary because the variable name immediately explains its purpose. If the code is well-written and variable or function names make sense, most comments can be safely omitted.
Use simple, understandable language
Sometimes we forget about obvious things. Comments should be understandable to other programmers. They should be simple and specific. For example:
// Function processing input data and returning output result
function processInput(inputData) {
// Here we perform various operations on input data,
// using different helper functions to manipulate them.
// We need to ensure that the final result is correct and meets expectations.
// Remember not to forget about implementation details
// and consider all edge cases.
// This is critical for the effective functioning of the function.
// We check if the input data is correct and if there are any errors.
// All exceptions are handled in this function,
// ensuring that the function does not unexpectedly terminate.
// Finally, we return the processed output data.
return processedData;
}
The above comment contains a lot of text but lacks specifics. It lacks specific information about the operations performed on the input data, the expected results, and any edge cases or problems that may arise. In other words, it's a lot of text that doesn't add much to the topic.
Comment on errors found in the code
Sometimes we add comments not only while writing code but also later, during its modification. And that makes sense. If we find an error in the code and manage to fix it, sometimes it's useful to explain this problem with a comment.
function isInternetExplorer() {
// Fixed behavior for Internet Explorer
// We use the navigator.userAgent method, which returns information about the browser.
// If the user agent contains the phrase "MSIE" or "Trident", it means we are using Internet Explorer.
return (navigator.userAgent.indexOf('MSIE') !== -1 || navigator.userAgent.indexOf('Trident') !== -1);
}
The comments in the above code explain why this code was created. They explain that it's necessary due to the different implementation of the function for the Internet Explorer browser.
Comment on incomplete implementation
Sometimes we need to commit code to a repository that has not been completed. In such a situation, it's worth clearly indicating that a particular function has not been fully implemented. Here's an example:
// TODO: Function for filtering even numbers in an array
function filterEvenNumbers(numbers) {
// This function is supposed to return a new array containing only even numbers
// from the passed array, but it has not yet been fully implemented.
// Code to be completed.
}
We have a function skeleton that, for some reason, has not yet been implemented fully. And we clearly indicate this with comments.
Comment on unusual code fragments
If you have any unusual implementations in your code, it's worth describing them with comments. Consider the following example:
// This function applies alternative methods of file reading for Internet Explorer browsers
// that do not support the FileReader interface. It uses the ActiveX method for file handling.
function readFile(file) {
if (isInternetExplorer()) {
// Code for handling files in Internet Explorer using ActiveX.
} else {
// Standard file handling using the FileReader interface.
}
}
In the code, we have an unusual implementation for an old browser. Here, using comments makes sense - they justify and explain our unusual code.
Summary
In the article, we described rules for writing good comments. We showed that comments should not duplicate code, should be written in simple, understandable language, and should be specific. Well-written comments are a valuable way to document code and greatly facilitate teamwork.