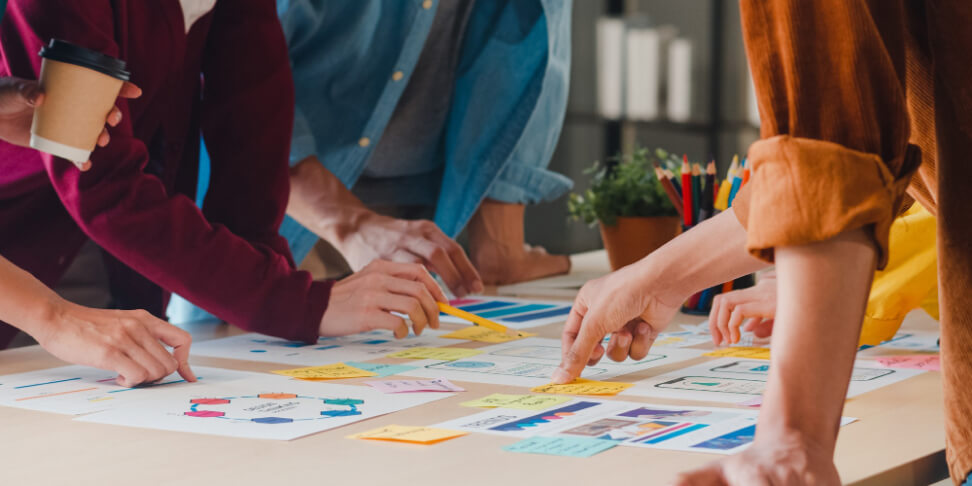
Autor: 27.07.2023
Introduction to Design Patterns
Design patterns are reusable solutions to common problems that occur in software design and development. They provide a set of proven solutions and best practices that can be applied to various software projects. Design patterns help in creating more flexible, maintainable, and scalable software systems.
Design patterns are not specific to any particular programming language or technology. Instead, they focus on the general principles of object-oriented design and programming. By using design patterns, developers can enhance code reusability, improve code organization, and promote better collaboration among team members.
Learning and applying design patterns require a solid understanding of object-oriented principles and programming concepts. It is important to choose the right pattern for a given situation and ensure that it aligns with the project requirements and goals.
Main categories of Design Patterns
There are various types of design patterns, classified into three main categories: creational, structural, and behavioral patterns. Creational patterns focus on object creation mechanisms, structural patterns deal with object composition and relationships, and behavioral patterns concentrate on the interaction between objects.
Common Patterns
Some commonly known design patterns include the Singleton pattern, Factory pattern, Observer pattern, and Strategy pattern, among others. Each pattern addresses a specific problem and provides a standardized way to solve it. By understanding and applying these patterns, developers can save time and effort by leveraging existing solutions instead of reinventing the wheel.
An example of a Design Pattern
Here is an example of implementing the Decorator pattern in Python. The Decorator pattern belongs to the group of structural patterns. Here is our code:
class Component:
def operation(self):
pass
# Concrete component
class ConcreteComponent(Component):
def operation(self):
print("Executing operation in ConcreteComponent")
# Decorator
class Decorator(Component):
def __init__(self, component):
self.component = component
def operation(self):
self.component.operation()
# Concrete decorator
class ConcreteDecoratorA(Decorator):
def operation(self):
super().operation()
self.additional_operation()
def additional_operation(self):
print("Executing additional operation in ConcreteDecoratorA")
# Another concrete decorator
class ConcreteDecoratorB(Decorator):
def operation(self):
super().operation()
self.other_operation()
def other_operation(self):
print("Executing other operation in ConcreteDecoratorB")
# Usage example
component = ConcreteComponent()
decorated_component = ConcreteDecoratorA(ConcreteDecoratorB(component))
decorated_component.operation()
In this example, we have a Component interface that defines the operations that can be performed. The ConcreteComponent class implements the Component interface and represents the core functionality.
The Decorator class, also implementing the Component interface, acts as a base class for concrete decorators. It maintains a reference to the wrapped component and delegates the operation to it.
The ConcreteDecoratorA and ConcreteDecoratorB classes inherit from the Decorator class and add their own behavior before or after calling the wrapped component's operation.
Finally, in the usage example, we create a ConcreteComponent object, and then we wrap it with multiple decorators (ConcreteDecoratorA and ConcreteDecoratorB). When calling the operation method on the decorated component, it triggers the execution of the additional operations defined in the decorators along with the core operation of the component.
The Decorator pattern allows us to dynamically add functionality to an object by wrapping it with one or more decorators. It provides a flexible alternative to subclassing for extending the behavior of individual objects.
Pros and cons of Design Patterns
Pros
Predictability: Design patterns are proven solutions that have been tested and applied in practice. As a result, one can expect that a solution based on a pattern will be effective and meet expectations.
Reusability: Design patterns promote code reuse. By implementing a pattern, we create a solution that can be used in various contexts and multiple times within a project or in other projects.
Facilitating Communication: Design patterns provide a common language and conceptual model that facilitates communication among members of a project team. This allows programmers and designers to easily discuss solutions and collaborate more effectively.
Flexibility and Scalability: Design patterns enable the design of flexible and scalable systems. By separating responsibilities and employing loose couplings between components, patterns allow for easy addition, removal, and modification of functionality without affecting the entire system.
Cons
Increased Complexity: Design patterns introduce an additional layer of abstraction and structure to the code, which can make it more complex for novice programmers to understand. Excessive use of patterns can lead to overly complicated and difficult-to-maintain code.
Increased Workload: Implementing design patterns requires time and effort. It involves understanding the pattern, its application in a specific context, and implementing it in the code. In some cases, especially for simple projects, using patterns may be an unnecessary overhead.
Potential for Misuse: Improper use of design patterns can result in overly complex code, increased dependencies between components, or excessive code duplication. Design patterns should be applied when they genuinely solve specific design problems and bring benefits, rather than just for the sake of using patterns.
No Universal Solutions: While design patterns provide solutions to common problems, there is no one-size-fits-all pattern. Each project has its specific requirements and context, which may require adapting patterns or applying other design techniques.
Summary
Design patterns are tools, not goals in themselves. Their application should be pragmatic and tailored to the individual needs and requirements of the project. Patterns are simply guides meant to assist in solving common programming problems.