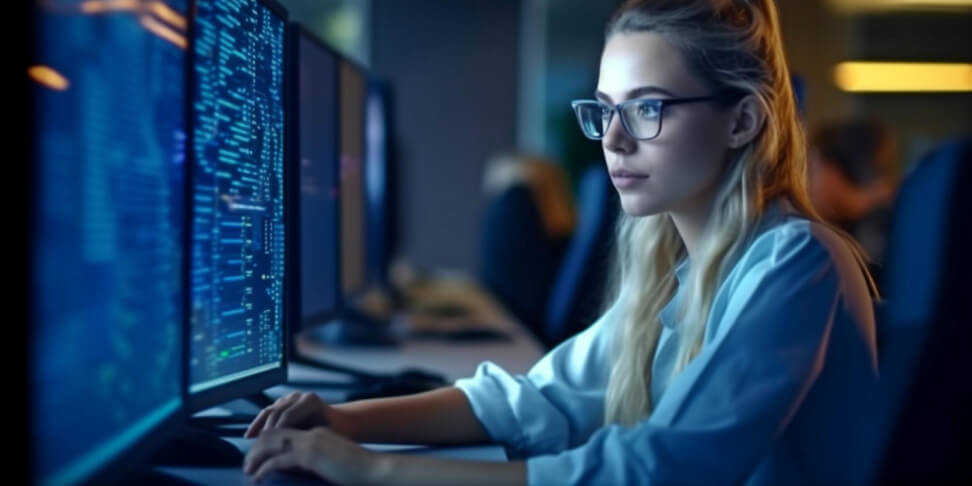
Autor: 16.01.2024
Java - incrementation and decrementation
Increment and decrement operations are relatively simple, but it's essential to pay attention to certain details to avoid future issues. In this article, we will explain the key concepts related to increment and decrement in the Java language.
Increment and Decrement
The technique known as incrementation is nothing more than increasing the value of a variable by 1. Look at a simple example:
int a = 1;
a = a + 1;
a += 1;
This can also be written in a concise form:
a++;
Decrementing is simply decreasing the value of a variable by 1:
int a = 1;
a = a - 1;
a -= 1;
This can also be written in a concise form:
a--;
Pre and Post Increment/Decrement
Now that you know what increment and decrement are, each of these operations can be either pre or post. What do these mysterious words mean? In increment and decrement, the word "pre" means that the ++ or -- signs are placed before the variable:
int a = 0;
++a;
--a;
On the other hand, the word "post" means that the ++ or -- signs are placed after the variable:
int a = 0;
a++;
a--;
Pre and post increment/decrement are associated with certain intricacies. Look at the example below; it will clarify many things:
public class Main {
public static void main(String[] args) {
int a = 10;
int b = 10;
int c = 10;
int d = 10;
System.out.println(a++);
System.out.println(++b);
System.out.println();
System.out.println(c--);
System.out.println(--d);
}
}
Result:
0
11
10
9
What happened here? You need to realize that post-increment (a++) will execute later. The println() function will first display the value of a, and only then its value will be increased by 1. The opposite situation occurs with pre-increment (++a). Pre-increment will execute earlier. The value of the variable will be increased by 1 first, and then the println() function will output the value. The situation is analogous for pre and post-decrement!