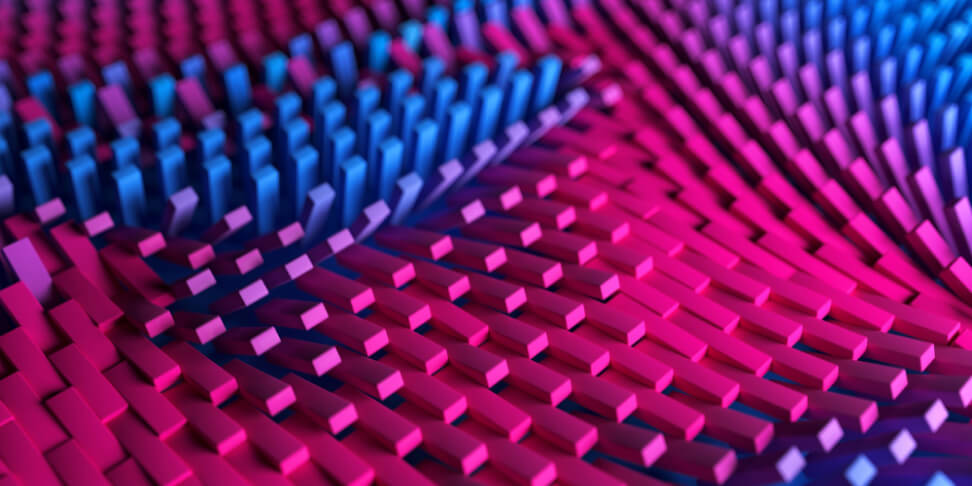
Autor: 12.06.2023
Java - introduction to multidimensional arrays
There is nothing difficult about multidimensional arrays. Read this short article and for yourself!
A simple example of a multidimensional array
Remember the game of battleships? A game board is very similar to a multidimensional array. We will show you how to declare such a board:
char[][] board;
Let's try to create a board consisting of 4 rows and 4 columns:
char[][] board = new char[4][4];
The board should be understood in this manner:
char[][] board = new char[row][column];
The array can also be initialized using the following technique. The size of the array will be calculated dynamically based on the number of elements. Doesn't a two-dimensional array remind you a bit of a battleship board? Take a look. We put ships on it to make it more clear.
public class Conversion {
public static void main(String[] args) {
char[][] board = {
{' ', ' ', ' ', ' '},
{'o', ' ', ' ', ' '},
{'o', ' ', ' ', 'o'},
{'o', ' ', ' ', ' '}
};
}
}
Let's look at the two-dimensional array above. We have the following ships available:
- 1 single-mast ship
- 1 tri-mast ship
How do we sink our single-mast ship? The table indexes are counted from zero. Our single-mast ship is in column 3 and row 2.
We can easily calculate its position ie:
board[2][3]; //namely 'o'
Let’s process the array with a loop
It looks pretty good. Do you remember program loops? Loops and arrays can cooperate with each other perfectly. Nothing changes here. We can display the whole board very neatly. Since we have a two-dimensional array we will have to use two loops (nested loops) . A nested loop is one that is inside the body of the other loop. Here is an example:
for(int i = 0; i < 5; i++) {
for(int j = 0; j < 5; j++) {
}
}
Let's use this knowledge to display our board:
public class Conversion {
public static void main(String[] args) {
char[][] board = {
{' ', ' ', ' ', ' '},
{'o', ' ', ' ', ' '},
{'o', ' ', ' ', 'o'},
{'o', ' ', ' ', ' '}
};
for(int i = 0; i < board.length; i++) {
for(int j = 0; j < board[i].length; j++) {
System.out.print(board[i][j]);
}
System.out.println();
}
}
}
The variable i is responsible for the row position, and the variable j is responsible for the column position of our game board. The nested loop is responsible for drawing the ships, and the surrounding loop is responsible for writing out new lines.
Here is the result of our work:
o
o o
o
Summary
Yup! That’s it. As you can see, there is nothing overly difficult when it comes to using multidimensional arrays in Java.
If you want to learn Java from the basic level and acquire the skills needed for a Junior Java Developer position, start your learning journey with the Career Path. The learning concludes with an exam that qualifies you to use the Certificate of Completion.