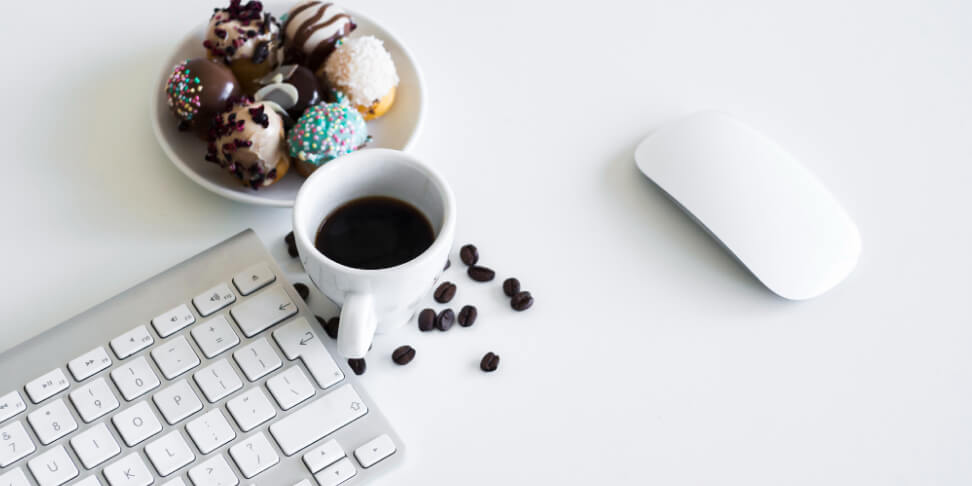
Autor: 05.04.2023
Java - primitive and object data types
Have you ever wondered, what is the difference between String and string? Have you ever gotten a compilation error because of using the wrong kind of data type? If so, here鈥檚 an article to help you get a grasp on the situation :)
Java has two kinds of data types: primitive and object data types. Just at first glance they can be differentiated pretty easily. A name of a primitive type begins with a lowercase letter:
int digit = 1;
A name of an object type starts with a capital letter:
String name = "Admin";
In practice, there are great differences in the way the two types function. We will go over them in a second.
Primitive types - what鈥檚 their thing?
Primitives are built into Java. This means you can use them straight from the start, no additional prep needed. You can simply declare a variable and decide what kind of data type it will be storing. These types are called the primitive types or just primitives.
Here鈥檚 a few examples:
int score = 23;
boolean finished = true;
double price = 23.99;
If you know Java fundamentals you may recognize the data types above. What can we say about them?
- Primitive types always have a value - they cannot be of null value.
- The name of the type starts with a lowercase letter.
- These types do not have their methods because they are not objects.
- These types do not have properties.
In Java there is a standard set of primitive types: char, boolean, byte, short, int, long, float and double. Generally we use them whenever we need to store a simple, singular value. A classic example of their usage could be a loop counter, or some simple functionality in a program that assumes the values of true or false.
What鈥檚 important, primitive types offer better efficiency in comparison to the object data types. This is tied into the mechanism of storing primitive type values - it鈥檚 way simpler than in object data type鈥檚 case.
Object data types - what鈥檚 their thing?
Object data types are used just like objects. Let鈥檚 kick off with an example:
String name = "Admin";
Here we have a variable named name that is of type String. Take notice that the name of the type itself begins with an uppercase letter.
Object data types can sometimes be called reference types. Why is that? It鈥檚 because here, we are dealing with a reference to a value. Our name variable is a reference to a String value. Such reference points to the location containing information about the value. It鈥檚 way different from how it works with primitives, where the values are stored directly in the variable.
What can be said about object data types?
- The name of the data type starts with an uppercase letter (e.g. String instead of string).
- These types are objects, and so - we have various methods and properties at our disposition.
- These types can be assigned null values.
The best way to present these characteristics is to use examples. Number one:
String name = null;
Null values can be used, whereas in the case of primitive types such operation would be impossible. What鈥檚 interesting is that null is the default value for object data types!
Another one:
String name = "Admin";
System.out.println(name.length());
We can access a variety of properties and methods. Here we have used the length() method from the String class - it would return the length of the text string (5 in this case).
When it comes to primitive types, we do not have access to this kind of content.
Custom object data types
The key characteristic of object data types is that we can create our own, custom, data types. We鈥檙e not limited to the default set only, every time you create a class, really you鈥檙e just creating a new data type. And everything checks out:
- A class name must start with an uppercase letter.
- We can create methods and fields in a class.
When to use primitive types
As we鈥檝e mentioned before, primitives are best when you need a singular value without any additional methods or properties. All types of loop counters, simple variables storing single values etc. The good thing here is the simplicity and efficiency stimming from the fact that values are stored directly in the variables themselves.
When to use object types
Object types are best to use when your data is complex. A good example would be some sort of class that represents a very complicated structure. A distinct majority of the programs written in Java are basically built upon multitudes of classes. Such classes have many methods and properties each.