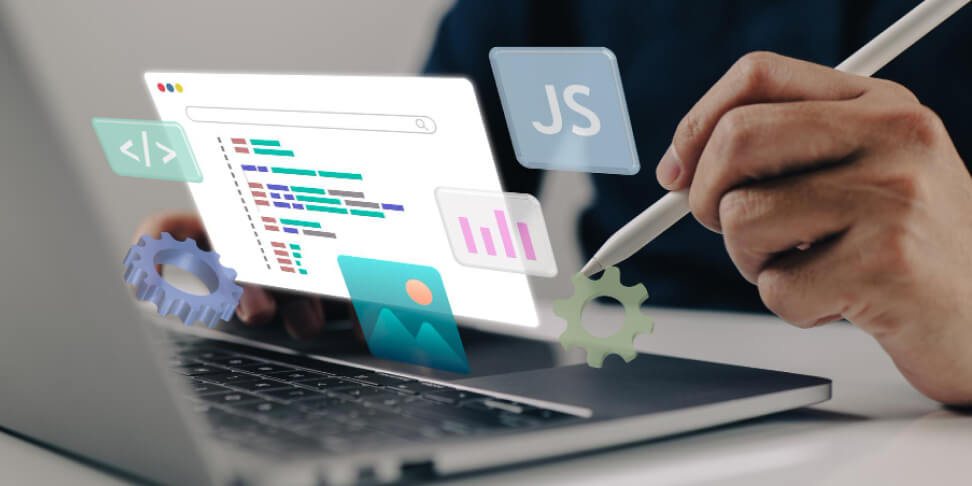
Autor: 07.06.2023
JavaScript - let, var variables and variable hoisting
In JavaScript you can declare variables by using the keywords var and let. Each of them has its own consequences , which you will learn more about in this very article.
Variable declaration var and hoisting
Take a look at this code example:
console.log(a);
var a = 1;
Notice how at first we are trying to address the variable and then, in the next line, we go ahead and declare it. What do you think is going to happen?
undefined
We’re getting an undefined, aka “value undefined”. JavaScript is pretty exceptional in that regard. In many other languages this situation would throw an error and the run of the application would be terminated.
The process seen in the example is what we call hoisting. In practice it basically means that the declarations are moved (hoisted) to the very top of the section. This is why JavaScript “knows” about our variable a despite it being declared below the place where we call it.
Now let's do the same example but using let:
console.log(a);
let a = 1;
This time we have a different example:
ReferenceError: a is not defined
Reference error - JavaScript knows nothing about our a variable. It’s easy to deduce that hoisting does not take effect (not directly at least) for variables declared using let.
In practice it’s best to simply declare your variables at the top of the code, no matter whether you use let or var.
Hoisting - let's dive deeper
We need to get back to something important:
console.log(a)
var a = 1;
Why are we getting undefined after running the code?
JavaScript uses hoisting for the declaration of a variable, but does not use it for the value assignment! So in our example, the part being hoisted is just the var a declaration. The assignment of value, a = 1, stays where it is. This is why, when we try to reference the a variable its value is undefined. The line below is where the value of 1 is assigned to the variable.
The code could also be written like this:
console.log(a)
var a;
a = 1;
The result would be exactly the same, namely undefined.
Variable scope - another piece of the puzzle
We could say that variables can be global or local. A global variable is one that can be accessed from anywhere. Let's say we have the following declaration at the top of our code:
let a = 1;
This is a variable that any given piece of our code can reference. It would seem it’s the perfect solution, but not necessarily. Why?
If every piece of our code can access this global variable, every piece of code can also modify it - and from there it’s extremely easy to create unexpected errors. The more complex the program you're writing, the greater the risk of your global variables being modified in a hard to predict way.
The solution
This is why in practice we often use variables which are limited to a certain scope. It’s a good habit because then we can be sure that other pieces of code will not affect how our function runs and will not modify our variable to an unpredictable value.
The var variable can either have the global scope or the scope of a function. The let variable can be global or have the scope of a block.
What’s a block? It’s any given piece of code which is closed on both sides with the curly braces.
Look at the example:
for (let i = 0; i <= 5; i++) {
console.log(i);
}
console.log(i);
This code results in our old friend, the ReferenceError error. The i variable is declared using let, so its limited to inside the loop block. Outside of it, the variable cannot be referenced!
The same loop, declared with var this time:
for (var i = 0; i <= 5; i++) {
console.log(i);
}
console.log(i);
In this case we can reference a variable from outside the loop block. Another difference between let and var.
Declaring variables with let is a more modern approach than using var. Its advantage is that variables like that are only limited to the block they are in - it minimizes our risk of overwriting the value on accident.
Summary
Every JavaScript programmer should know the differences between the var and let declarations. This knowledge is key in everyday work. You also need to understand the true essence of variable hoisting and the extremely important concept of scopes.
If you want to learn JavaScript and gain the skills needed to work as a JavaScript Developer, start the journey with our Career Path. The whole process ends with an Exam, which will grant you the Specialist Certificate!