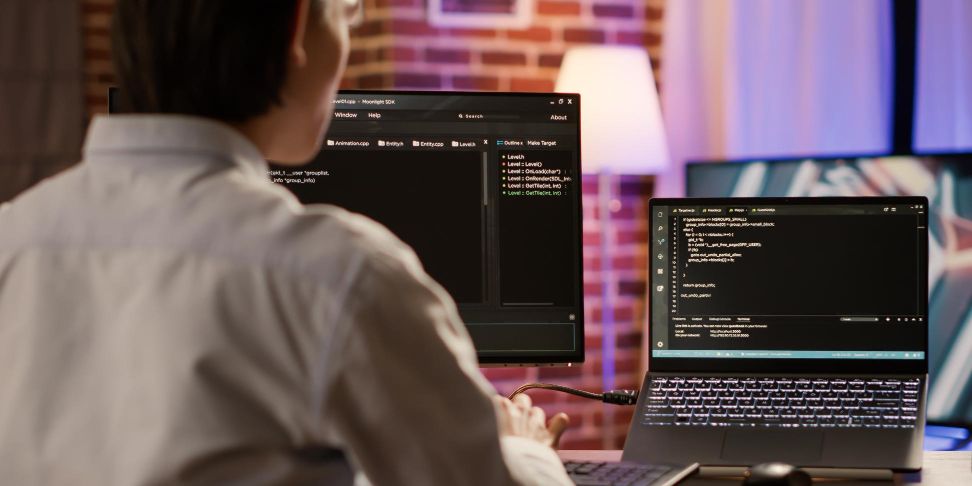
Autor: 29.09.2023
NumPy - What Is It
NumPy is a popular Python library for performing numerical and scientific computations. The central component of the library is functions for working with arrays of numbers. In this article, we will show you how to get started with this incredibly useful tool.
Installation and First Steps
To work with the NumPy library, you need a local installation of the Python language. So, at this stage, we assume that Python is correctly installed and configured on your system. If you are using the PIP package manager, installing the library is straightforward:
pip install numpy
Simply enter the above command in the terminal. If the library has been installed correctly, you can start using it. You may recall that you need to import each library into your Python code file first. As a reminder, this is done as follows:
import numpy as np
We import the entire contents of the numpy library as 'np,' which makes it easy to reference individual functions.
Creating Arrays
As mentioned at the beginning, the fundamental element is arrays of numbers on which we can perform various operations. Let's start by creating some sample arrays:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
We used the array() function to build the first array. We passed a regular Python list as an argument. This list is effectively converted into a NumPy array. Why do we do this? Because arrays are the main data structure on which operations are performed in NumPy. Additionally, they provide better performance than standard Python lists.
Other Examples of Arrays
We also have other interesting ways to create arrays:
arrayOne = np.ones(5)
arrayZero = np.zeros(5)
Here, we create two arrays containing five ones and five zeros, respectively. This is what they look like in the console:
[1. 1. 1. 1. 1.]
[0. 0. 0. 0. 0.]
Now, let's generate an array with a specified range of numbers:
arrayRange = np.arange(1, 10)
The result looks like this:
[1 2 3 4 5 6 7 8 9]
This is just the beginning of what's possible. If you ever need more information, you can always consult the NumPy documentation
Arithmetic Operations
Creating arrays by itself doesn't give us much. The most important part is the operations we can perform on these arrays. Let's start with simple arithmetic operations:
import numpy as np
array1 = np.array([1, 2, 5, 4])
array2 = np.array([5, 6, 7, 8])
sum = array1 + array2
print("Sum: ", sum)
difference = array1 - array2
print("Difference: ", difference)
In the above code, we created two arrays of numbers and performed two operations: addition and subtraction. Here's what the result looks like:
Sum: [ 6 8 12 12]
Difference: [-4 -4 -2 -4]
Notice how these operations work. In the case of addition, we add values from two arrays: 1+ 5, 2 + 6, 5 +7, 4 + 8. Similarly, it works for subtraction: 1 - 5, 2 - 6, 5 - 7, 4 - 8. If you look at the results, everything should be clear.
Logical Operations
Now, let's perform some simple comparisons. Here's our code:
import numpy as np
arr1 = np.array([1, 2])
arr2 = np.array([5, 4])
result = arr1 > arr2
print("arr1 > arr2:", result)
result = arr1 <= arr2
print("arr1 <= arr2:", result)
The result looks like this:
arr1 > arr2: [False False]
arr1 <= arr2: [True True]
Why these results? Comparisons are made pairwise. First, we check if the values from arr1 are greater than the values from arr2. That is, 1 > 5, 2 > 4. Both comparisons are false, so the result is [False False]. In the second example, we check if the values from arr1 are less than or equal to the values from arr2. That is, 1 <= 5, 2 <= 4. Both comparisons are true.
Statistics and NumPy
Another interesting example involves various functions for statistical calculations:
import numpy as np
data = np.array([1, 6, 2])
mean = np.mean(data)
median = np.median(data)
max_value = np.max(data)
min_value = np.min(data)
print("mean: ", mean)
print("median: ", median)
print("max_value: ", max_value)
print("min_value: ", min_value)
We are working with a simple array and calculating the following values: mean, median, maximum value, minimum value. The result looks like this:
mean: 3.0
median: 2.0
max_value: 6
min_value: 1
As you can see, we have more examples of simple but very useful NumPy functions.
Summary and Next Steps
In this article, for obvious reasons, we could only present a very narrow slice of what NumPy offers. This library is a fundamental tool for countless engineers, scientists, and programmers. It's really worth exploring it further if you work in fields related to numerical computations.