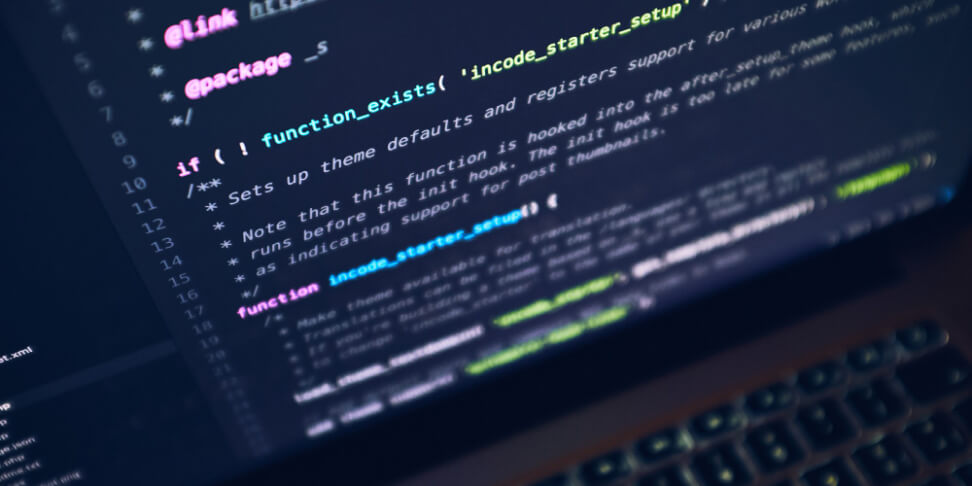
Autor: 08.12.2022
Object-Oriented Programming - what’s it all about?
Object-Oriented Programming (a.k.a. OOP) is a programming paradigm - it’s a way you can approach writing code.
In OOP the program’s structure is based on objects that communicate with one another. Imagine for a second you are writing an application that will allow you to create and publish articles. What objects would you need to achieve that?
First thing you’re going to need for sure is an object to represent an article and an object for an author. Then, you may also need objects for articles’ categories and whatnot. In reality, in fully developed applications there could be tens, or even hundreds, of objects.
Our final application will simply be multiple different objects put together to communicate and work together towards a desired common goal.
Thanks to this approach we are able to imitate objects from the real world in-code. Each element of the program could be imagined as a certain, well - object.
Concepts
Let’s talk a little more about objects. Each object has its own traits that we often call attributes. So an article object would have attributes like the title, content or creation date. An author object could have attributes like the name, last name or email address.
Another important concept is the object’s behaviors, which in OOP are often called the methods. Our article object could contain behaviors like: create, publish or edit.
As you can see it’s very much not rocket science :) All you need to do is to think with objects. Every part of the program you need to imagine as an object that has its attributes and methods.
Classes and instances
Most of the object-oriented programming languages are based on the so-called classes and instances. A class is a template, according to which instances can be created, instances being actual objects. Let’s take an example again.
Let’s say “Acticle” is a class, so a template to create an instance. “Introduction to OOP” would be an instance, or an object, of the Article class.
Take a look at the example class:
Article
attributes
title
content
creation_date
behaviors (methods)
create()
publish()
edit()
Here we have a class named Article that defines its attributes and behaviors. Each instance (object) of this class will have access to the attributes and behaviors - so each article here will have a title, contents etc. Each article will be able to be published, edited etc.
In the same way, you can create more classes for your application. See it as an exercise and think about what other classes might also be needed. What attributes and methods would they have? The OOP filosophy really only comes down to getting the classes right.
Advantages
Throughout the years object-oriented coding was the dominating approach to programming. In many situations that is the most intuitive way to look at the problem of designing an app’s architecture - the way we split up the system into objects reflects our natural thought process. Often we have a tendency to describe the world as objects that always have their own traits or descriptors.
- Object-oriented programming allows for splitting up the complexity of systems into smaller, easier to grasp elements.
- OOP lets the code be split into modules, which allows for reusing the same code pieces multiple times.
- OOP allows for standardization, which in turn makes teamwork easier.
Disadvantages
To avoid being biased it’s worth mentioning some flaws of OOP too.
Some programs are quite simple - they only perform a couple of not-that-complex operations. In such cases implementing the object-oriented approach can be a bit of an overkill. No sense trying to design these elaborate classes if our application is just a few simple actions.
For OOP to hold any water in a given project, its implementation has to have some basis to be there. It’s not difficult to suddenly find yourself in this “form over substance” trap. If your code is overly complicated, hard to maintain and/or susceptible to errors and mistakes, you might just be another victim of the aforementioned conundrum.
There are situations that naturally warrant usage of object-oriented programming, designing user interfaces (UI) for example. There are also moments when the situation would benefit from using OOP. Just be thoughtful about where you've just learned and measure your pros and cons.
Practice
The concept of OOP started out in late 60’s. As of now there are a lot of languages that allow for the object-oriented approach.
The most popular of them are Java, C#, C++, JavaScript or Python. The language itself and which you choose for your project is a side-issue. Object-oriented programming has some rules and principles of its own and they are what is the most important here. These mechanics are mostly universal and can be applied to many languages.