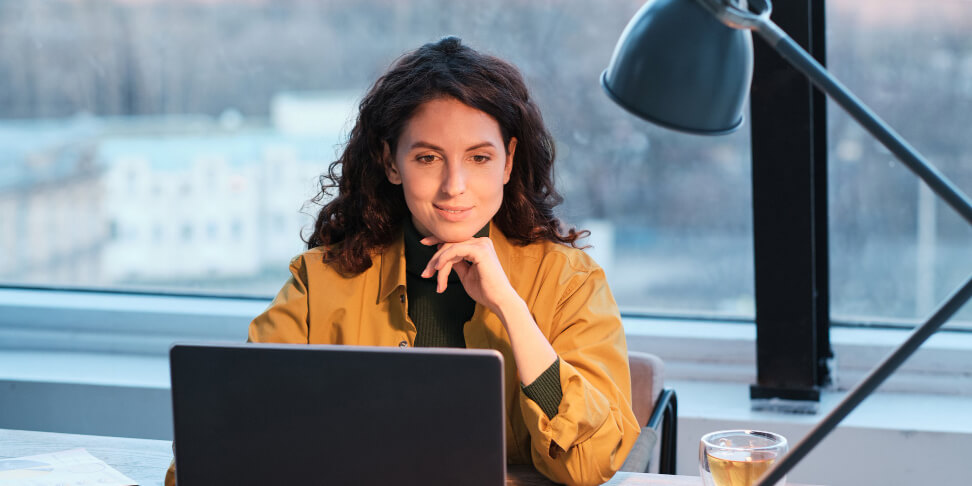
Autor: 30.03.2023
PHP - when to use the json_encode() function
PHP is a language that is mainly used for writing web applications. Such applications often exchange data in JSON format. PHP has a couple of useful built-in functions for working with JSON data. We will take a look at them in this article.
JSON - what’s that?
Let’s start with the basics - what is JSON? It is a very common data exchange format, used in numerous web applications. Its name stands for JavaScript Object Notation. Contrary to its name, it can be used by many programming languages - isn’t limited to JavaScript. It’s a very common and very universal data format.
Thanks to JSON, various web apps can talk to each other, and they can easily exchange data. That’s why many programming languages have ready-made functions for working with JSON data. And PHP is no exception - it comes with a very useful function named json_encode().
A basic example of json_encode()
Let’s imagine that we are building a web application that sends data as JSON. Our example data will be represented by this simple associative array:
<?php
$vals = array('a' => 1, 'b' => 2, 'c' => 3);
?>
We need to convert it into JSON. It's a very straightforward operation, as you will see in a moment. Take a look at this code snippet:
<?php
$vals = array('a' => 1, 'b' => 2, 'c' => 3);
echo json_encode($vals);
?>
We used a json_encode() function to…encode our data into JSON. Yep! That’s it! Here is the final result:
{"a":1,"b":2,"c":3}
We got a properly formatted JSON string. It has three key-value pairs. That’s the most basic example we can get.
Let’s reverse the operation - json_decode()
Now we will try to perform a reverse of the above operation. We want to convert JSON data into a PHP object or associative array. That’s a very common scenario: we get some data from an external source and we need to process it in some way. We will use a built-in json_decode() function for this purpose. Take a look at the example:
<?php
$json = '{"a":1,"b":2,"c":3}';
print_r(json_decode($json));
?>
We got a JSON string assigned to the $json variable. We used a json_decode() function to convert the data into a PHP object. Here is the result:
stdClass Object
(
[a] => 1
[b] => 2
[c] => 3
)
So we got a basic object with key-value pairs. As simple as it gets. We can also convert a JSON string into an associative array:
print_r(json_decode($json, true));
We added a second param to the json_decode() function. Its value is true - so the data will be converted into an associative array. And here is the final result:
Array
(
[a] => 1
[b] => 2
[c] => 3
)
We can easily process data like this in our PHP application.
Summary
Using the functions mentioned in this article, you can freely convert the data. Use the json_encode() to convert the data into a JSON string. Use the json_decode() function to convert a JSON string into an object or associative array.