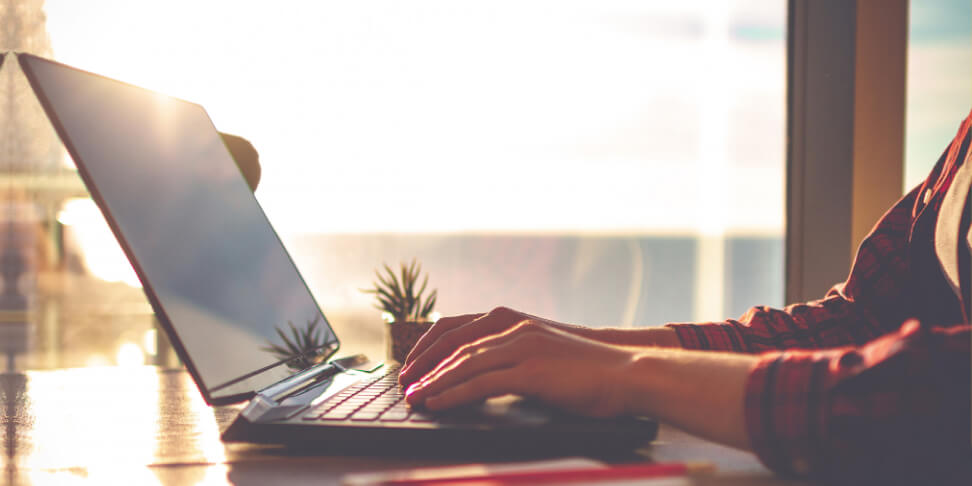
Autor: 10.03.2023
What is programming, exactly
In today’s world of modern technologies, programming holds a very important place. Without it, many things essential in our lives would simply never come to exist.
Programming is the art of writing instructions that tell the computer clearly what to do and when to do it. There are hundreds of programming languages - some well-known, others with very specific niches. Most of them however, are based on the same overall set of rules. Programming constantly reuses a few of the same core concepts.
Said concepts could be compiled into a list:
- Syntax
- Variables
- Data types
- Data structures
- Operators
- Conditional statements
- Iteration
Syntax
Syntax is a set of rules used for writing the program’s code. Every language has its own distinct syntax. It’s a very important element of the whole process - you must strictly adhere to the rules. Computers require clear instructions from us, everything has to be written in an unambiguous way.
Let's take a look at this simple program written in Python:
print('Hello, world!')
This program prints out a short welcome message and is written using Python-typical syntax rules. If not for them, the computer wouldn't know what to do with the code.
That’s why learning the syntax is a fundamental step you need to take while learning programming.
Variables
A big part of programming consists of data processing, and variables are what allows us to store information in a computer's memory. A variable is a fragment of memory that has its own name, and that you can assign data to. When it comes to ways to utilize variables, the sky’s the limit. Our program could have a variable that stores the current date, a username, a number of an order just to name a few - it could be literally anything.
Now, here’s a simple example:
username = "Admin"
In most programming languages working with variables consists of naming the variable and assigning a value to it. In the example above, we've created a variable named username and we've assigned the value Admin to it. If, let's say, we wanted to check the current username, we would just have to check what value is assigned to the variable. We can also modify the value at any point in the program.
Data types
Now that we’re at the topic of data, it’d be worth mentioning that there are multiple distinct types of values you can assign to variables. Here are a few examples:
username = "John"
score = 1
price = 12.5
finished = True
Above we’ve created four variables - each containing a different type of value. The username value stores text, score stores an integer, price - a floating-point value, and finished stores a boolean value (True or False).
Often there’s a situation that calls for the programmer to foresee what data type will be stored in a given variable. That’s why you need to have a good insight into the properties of the most important data types in the programming language you’re in at the moment.
Data structures
Data structures are sets of values that adhere to certain rules. A simple example of such structure is a list, look at the example:
users = ["Ted", "John", "Alice"]
We have created a list of three names - now we have one variable that stores many values. Thanks to data structures like this one we have the ability to group logically associated values.
Groups of values like the one above in many programming languages are called arrays. There are others, more complicated structures too, like: stacks, queues, trees etc.
All the structures allow you to organize and keep the data in your program’s code orderly, and this is exactly why they play such an essential role in programming.
Operations
All the data we store is ultimately for us to be able to perform operations on them. To achieve this we would use the so-called operators.
Arithmetic operators can often be seen used in programming. They are the well-known and loved operations like addition (+), subtraction (-), division (/) and multiplication (*). We also have operators that allow compare values:
== checks whether or not two values are equal
> checks whether or not the value on the left is greater than the one on the right
etc.
Additionally there are also the logical operators. Take a look:
2 == 2 and 4 == 4
2 == 2 or 3 == 4
not (2 == 2)
The and operator demands that both parts of the statement be true. The or operator demands that at least one part of the statement be true, and the not operator performs a negation - the statement will be true if the condition is NOT true.
Conditional statements
Thanks to conditional statements, our program can make decisions. There are endless examples:
- if the price exceeds $100, apply a discount,
- if the used passed a wrong password, show information about the error,
- if the game has been finished, show results.
In most of the popular programming languages, we implement such mechanisms with conditional statements. They can be described with a simple schema:
IF (CONDITION FULFILLED)
PERFORM AN OPERATION
The above operation will only be performed if the condition is true. Sometimes there can also be a more expanded version of the thing above:
IF (CONDITION FULFILLED)
PERFORM AN OPERATION
OTHERWISE
PERFORM A DIFFERENT OPERATION
Now we’ve got an alternative: either one or the other operation will be performed. It all depends on whether the condition is fulfilled or not.
Iteration
There’s often a need in programming to repeat actions. That is actually a thing computers can do very well. Imagine you have to generate a list of numbers, 1 to 10.000 - if you were to do it manually, we would probably give out at around 30. Luckily, a computer can do the task for us in a fraction of a second.
And iteration is exactly that - the repetition of a task. In practice we perform this operation with a loop. Take a look at how a general schema of such a loop could look like:
UNTIL (CONDITION FULFILLED)
REPEAT THIS ACTION
Here’s how it works: we repeat an action until the condition is fulfilled. At one point a value will be reached that doesn’t fulfill the condition anymore - and this is the moment our loop breaks. Back to the example:
1. Starting with 1 increment the value by 1.
2. Check the value continuously.
3. If said value reaches 10.000, break the loop.
That’s how you could describe a loop that generates numbers from 1 to 10.000.
Summary
The concepts we’ve gone over in this article are what the absolute fundamentals of programming consist of. They are all universal and can be found in almost every language. It’s necessary for everyone beginning their programming journey to be familiar with them.
Almost every program will use variables and various data types. Every program must be written according to the language’s rules and syntax. Oftentimes using data structures, different types of operators or introducing conditional statements can't be avoided - all this is required knowledge in any branch of programming you might find yourself in.