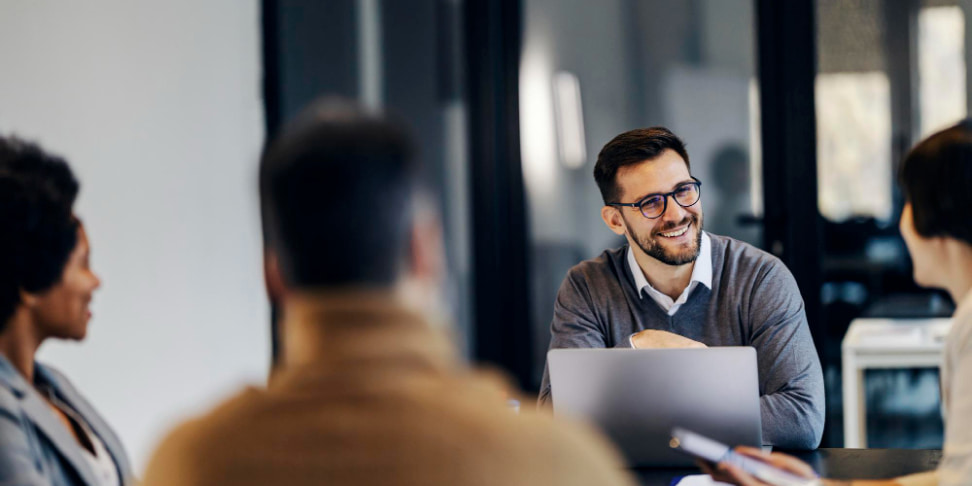
Autor: 29.08.2023
Python - example interview questions
Embarking on a career as a Python Developer is an exciting journey, but navigating the interview process can be a challenge. In this article, we've curated a set of real-world Python interview questions that cover a spectrum of topics. Use them as a guide to see what to expect during interviews.
What are the differences between tuples and lists?
Tuples and lists are two separate data types in Python. Both are used to store collections of elements, but they have some differences.
Example: if we want to store the names of a few people, we could use either tuples or lists. An example of how to use both:
# example tuple
persons = ('Emily', 'Mary', 'Linda')
# example list
persons = ['Emily', 'Mary', 'Linda']
In the code above we create both a tuple and a list containing the names of three people. The tuple is created using parenthesis, and a list - with square brackets.
Main differences between tuples and lists in Python are:
- Tuples are immutable, which means you can't modify them after they are created. Lists are mutable, which means you can add to them, delete from them and modify their elements.
- Tuples take up less memory space than lists do.
- Tuples are faster to process than lists are.
- Tuples can be used as keys in dictionaries, and lists cannot.
What is PEP8?
PEP8 (Python Enhancement Proposal 8) is a document containing recommendations related to the style of coding in Python. PEP8 aims to standardize the appearance and readability of code, and to make it easier for programmers to understand each other’s code and cooperate.
Here are a few basic pieces of advice from PEP8:
- Indentation: Use four spaces as your level of indentation (with no tabulation).
- Spaces around operators: Add spaces before and after operators (e.g. =, +, -, *, /).
- Line length: Try to keep your lines shorter than 80 characters. In case of longer lines, you can split them up with the "\" operator or put them inside parentheses.
- Naming: Use descriptive names for variables, functions and classes, as per the snake_case convention, and PascalCase for classes.
- Spacing: Add spaces between operators, after commas and colons, but not at the end of the line.
- Comments: Add comments to explain otherwise complicated pieces of code, but try to write readable and self-documenting code.
Example of code adhering to PEP8:
def calculate_sum(a, b):
result = a + b
return result
Example of code disregarding PEP8:
def calculateSum(a,b):
result=a+b
return result
In the second example you can see the lack of spaces around operators and incorrect naming of functions.
Adhering to PEP8 helps maintain cohesion in your Python code, improving its readability and understanding - for you, as well as other developers.
What are the differences between local and global variables in Python?
In Python we have the option to define local and global variables. Local ones are only accessible from inside the block of code, e.g. a function, whereas global variables are accessible from anywhere in the program.
x = 12
def fun():
y = 10
print("x = " + str(x))
print("y = " + str(y))
fun()
print(x) # global / ok
# print(y) # local / error
In the above example, the variable y is a local one. Because it was defined inside a function, it can only be used there.
And the result:
x = 12
y = 10
12
In Python it’s worth remembering the difference between local and global variables. Local variables are useful when you want to cut the access to certain pieces of data, so that it can only be reached from inside the block of code it’s in. Global variables, on the other hand, are accessible in the entire scope of the program, and can be used by various functions.
What can be a key in a dictionary?
A dictionary in Python is a data structure, which stores pairs of key-values. Keys in dictionaries need to be unique and immutable, which means that they can't be modified after creating the dictionary. Keys can be of various types, like numbers, text strings or tuples.
Example:
dictionary = {'key1': 'value1', 'key2': 'value2'} # create dict
print(dictionary['key1']) # get value
dictionary['key1'] = 'value...' # update value
dictionary['key4'] = 'value4' # add value
del dictionary['key2'] # delete value
print(dictionary) # print dictionary
In the code above we presented various operations on dictionaries. We can add new pairs of key-value, modify the values based on its key and delete the key-value pairs based on the key.
And the result:
value1
{'key1': 'value...', 'key4': 'value4'}
What is PYTHONPATH?
PYTHONPATH is an environment variable in Python that determines what places in memory the Python interpreter will look for modules and packages.
When we import a module or a package in Python, the interpreter has to find its location. It looks for these elements in a few standard places, like the built-in modules, the current catalog path and the paths specified in the PYTHONPATH variable.
The PYTHONPATH variable is a list of paths separated by a path character (e.g. a colon in Unix/Linux systems, or a semicolon in Windows). It can contain paths to catalogs that contain the modules and packages that we want to use in our program.
If the PYTHONPATH variable is set, the Python interpreter will search through these paths to find the modules and packages to import.
Example:
export PYTHONPATH="/path/to/my/modules:/another/path"
In the example above we set the PYTHONPATH variable to be: /path/to/my/modules and /another/path. The Python interpreter will also look for modules and packages there.
The PYTHONPATH variable is useful when you want to have control over where the Python interpreter is to look for modules and packages. It can be especially handy when you have your own modules, defined in other locations than the standard ones.
Summary and the next steps
The questions in this article are intended to show you what you can expect in a job interview. Treat them as a guideline for the expected level of your skills.
Do you want to prepare well for the interview? Take advantage of the Python- Interview Assignments course. It contains dozens of similar questions. Each question in the course is discussed from both a theoretical and practical perspective.