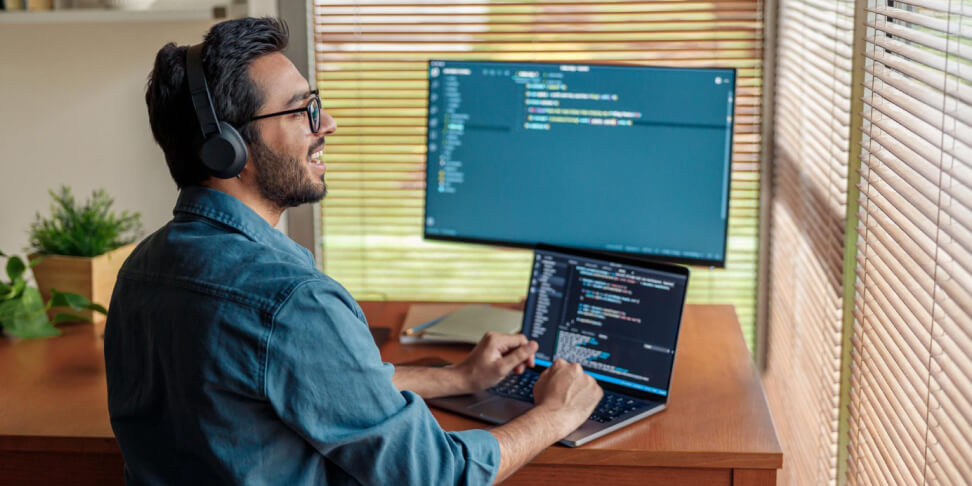
Autor: 25.01.2024
Python - How to Use the Console
The Python console is an interactive tool that allows you to write and execute code. It's a great way to learn the basics of Python and test short code snippets. In this article, we'll discuss the fundamental techniques of working with the Python console.
Installation and First Run
Before you can run Python on your computer, you need to install it first. Assuming Python has been properly set up on your computer, let's proceed.
To start working with the console, enter the command python after launching it. This will initiate the interactive Python interpreter. You should see something like this:

This view indicates that the Python interpreter has been successfully started. Now you can enter commands and Python code. Simply type a command and press Enter to execute it.
First Example - Hello World
Let's begin with a standard programming example:
>>> print("Hello, World!")
Hello, World!
Using the print() command, we displayed the text "Hello, World!" on the screen. Almost every beginner programmer starts with something similar. In the above example, you can see both the code we entered and its result.
Additional Examples
With the interactive interpreter, you can do a lot. You can perform arithmetic operations, create your own functions, and more. If you want to exit the console, just type the exit() command. Now, let's look at some of the things you can do in the console.
Arithmetic Operations
>>> 2 + 3 # Addition
5
>>> 5 * 4 # Multiplication
20
>>> 10 / 2 # Division
5.0
>>> 7 - 5 # Subtraction
2
In the Python console, you can perform standard arithmetic operations. Python acts like a calculator, allowing you to perform addition (+), multiplication (*), division (/), and subtraction (-) operations.
Working with Variables
>>> x = 10 # Assigning a value to a variable
>>> y = 5
>>> result = x + y # Operations on variables
>>> result
15
Python allows you to store values in variables. You can assign values to variables (e.g., x = 10) and perform operations on them.
Strings
>>> text = "Hello, World!" # Assigning text to a variable
>>> print(text) # Displaying text
Hello, World!
>>> len(text) # Length of the text
15
In Python, text is represented as strings. You can perform various operations on them, such as displaying (print) or checking the length (len).
Lists
>>> my_list = [1, 2, 3, 4, 5] # Creating a list
>>> my_list[0] # Accessing list elements
1
>>> my_list.append(6) # Adding an element to the list
>>> my_list
[1, 2, 3, 4, 5, 6]
Lists in Python are collections of elements stored in a specific order. You can add, remove, and modify their elements.
Conditional Statements
>>> number = 15
>>> if number > 10:
... print("The number is greater than 10")
...
The number is greater than 10
Conditional statements allow you to perform certain actions only when a specific condition is met. In this case, if the number is greater than 10, a message will be displayed.
Functions
>>> def add(a, b): # Function definition
... return a + b
...
>>> add(3, 7) # Function call
10
Functions allow you to group code for reuse. In this example, the add function takes two arguments and returns their sum.
Summary
In this article, we've only scratched the surface. We encourage you to experiment with the Python console on your own – it's a great way to master the basic concepts and mechanisms of the language.