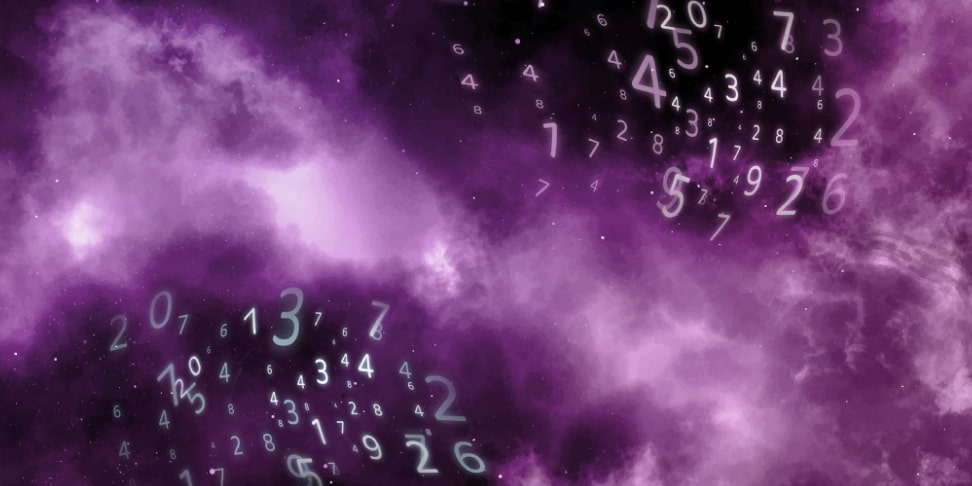
Autor: 19.07.2023
What is serialization?
In this article, we will explain the concepts of serialization and deserialization in programming.
How it works?
Serialization is the process of transforming an object into a sequence of bytes that can be saved to a file or transmitted over a network. When an object is serialized, it can be stored on disk as a file or transmitted over the network as a stream of bytes. Later, to restore the original object, we can perform the reverse process called deserialization, which involves reading the sequence of bytes and reconstructing the original object or data structure.
Serialization is useful in cases where we want to save the state of an object or data structure in order to later restore it or transmit it to a different location. It is commonly used in networked applications where objects need to be transferred between a client and a server.
Basic workflow
We have a simple workflow here:
- Object serialization
- Object transmission
- Object reading (deserialization)
First, we serialize the object, which means converting it into a serialized form, such as a byte stream, JSON, or XML representation. This serialized form contains the necessary data and information about the object's structure.
Next, we transmit the serialized object. This can involve sending it over a network to another location or storing it as a file on disk.
Finally, to retrieve the original object, we perform the deserialization process. This involves reading the serialized data and reconstructing the object or data structure from it. Deserialization restores the object to its original state, allowing us to work with it as if it had never been serialized.
This workflow enables us to transfer objects between different systems or store them persistently for later use.
Serialization - use cases
There are several typical cases where the benefits of serialization can be utilized.
Saving state
We can serialize objects that store the state of our application, such as user sessions, shopping carts, user preferences, and more. Serialization allows us to save these states in a database or on disk, so that we can restore them in the future.
Sending data over network
When we want to send data over the network to another computer or application, we can serialize it and transmit it in a format that is more compatible with network protocols, such as JSON or XML. On the receiving end, the recipient can read the data and reconstruct the object or data structure.
Saving data to database
Often, we want to store data in a database. For example, if we have objects representing users in our application, we can serialize them and save them as records in a database. Later on, we can read these records, deserialize them, and reconstruct user objects.
Saving data to a file
Serialization allows us to save data to files on a hard drive. For example, we could serialize a data structure to a text or a binary file, in order to read the data later on and recreate the object or structure.
Things to consider
There are certain issues that can arise from improperly implemented serialization. As always, experience and a proper approach to code design are crucial.
Security
Serialization can pose potential security risks. If serialized data is transmitted over a network or stored on external devices, it can be vulnerable to attacks.
Performance
The process of serialization and deserialization can be time-consuming, especially for large objects or data structures. If an application frequently utilizes serialization, it can impact the system's performance.
Complexity
If the data structure is complex or contains dependencies between objects, the serialization process can be challenging. This adds further challenges for programmers working on the code.
Serialization itself is an incredibly useful tool. However, like any other tool, it needs to be used wisely.
Implementing serialization
Implementing serialization in practice depends on the programming language we are using. Many languages provide interfaces, libraries, and other tools to support serialization implementation. You can find such tools in languages like Python, Java, or C#.
Summary
Serialization is a useful tool for saving the state of objects or data structures for later restoration or transmission. By serializing objects or data structures, we can convert them into a format that can be stored, transmitted, or persisted. This serialized form captures the necessary information to reconstruct the original object or data structure.