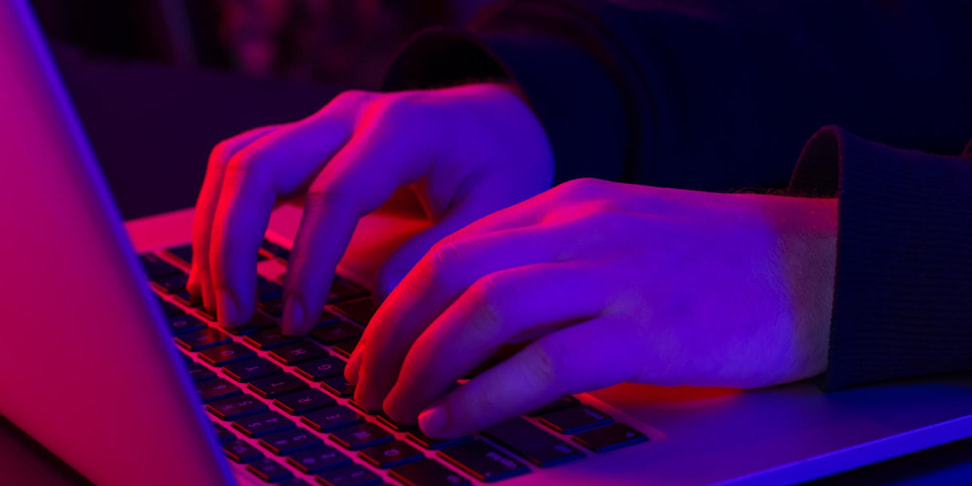
Autor: 28.09.2023
C# - Sample Interview Questions
C# is a highly popular programming language. Its versatility and universality make it a common choice among programmers for developing various projects. In this article, we will take a look at some sample interview questions related to the C# language. We hope these questions will help you gain a better understanding of industry expectations and increase your confidence during your interview preparations.
10 Sample Questions
Take a look at the following questions. Test your knowledge of the C# language.
Generic Classes
What is the purpose of using generic classes in the C# language?
A) Facilitating the creation of variables in the program
B) Allowing the storage of different data types in one class
C) Ensuring better program performance
D) Enabling the dynamic creation of objects
Correct answer: B. The main purpose of generic classes is to allow the storage of different data types in one class.
Generic Interfaces
What is a generic interface?
A) An interface that can only accept numeric data types.
B) An interface that can accept any data types.
C) An interface that can only accept text types.
D) An interface that can only accept arrays.
Correct answer: B. The primary goal of generics (including generic interfaces) is their universality, allowing the creation of structures that can accept any data type.
Enums
How is an enumeration type defined in the C# language?
A) enum Type;
B) enum Type() {};
C) enum Type = {value1, value2};
D) enum Type {value1, value2};
Correct answer: D. This is the correct way to define an enumeration type, with two values.
Math Class
What will be the result of the following code?
double number = 9.8;
double roundedNumber = Math.Round(number, 0);
Console.WriteLine(roundedNumber);
A) 9
B) 9.8
C) 10
D) 8.9
Correct answer: C. We use the Round() method from the Math class, which rounds the given value to the nearest integer.
Delegates
What is the role of the Predicate delegate in C#?
A) Performing mathematical operations on boolean variables.
B) Filtering data based on a specific condition.
C) Storing a list of primitive values.
D) Facilitating the creation of new data types.
Correct answer: B. The main use of the Predicate delegate is data filtering.
Lists in C#
How do you add an element to a list in C#?
A) list.Update(element);
B) list.Remove(element);
C) list.Clear();
D) list.Add(element);
Correct answer: D. New elements are added using the Add() method.
Stacks
What are the two fundamental operations that can be performed on a stack?
A) Push and Pop
B) Add and Remove
C) Insert and Delete
D) Enqueue and Dequeue
Correct answer: A. Stacks have their own specifics: the element added last (on top) is the first to be removed. In practice, we refer to these operations as Push and Pop.
Dictionaries in C#
What is the result of executing the following code?
Dictionary myDictionary = new Dictionary();
myDictionary.Add("apple", 3);
myDictionary.Add("banana", 5);
myDictionary.Add("orange", 2);
Console.WriteLine(myDictionary["banana"]);
A) "apple"
B) "banana"
C) "orange"
D) 5
Correct answer: D. Here, we have a dictionary, and we're trying to access the value at the key ["banana"], which is 5.
Exceptions
Which block is responsible for catching an exception?
A) try
B) catch
C) finally
Correct answer: B. Exceptions are caught in the catch block.
LINQ - Select
Look at the code below and answer: What values will be the result of the query?
var numbers = new[] { 1, 2, 3 };
var query = numbers
.Select(x => x + 2)
A) 1, 2, 3
B) 3, 2, 3
C) 3, 4, 5
Correct answer: C. We use the LINQ Select query and add 2 to each element.