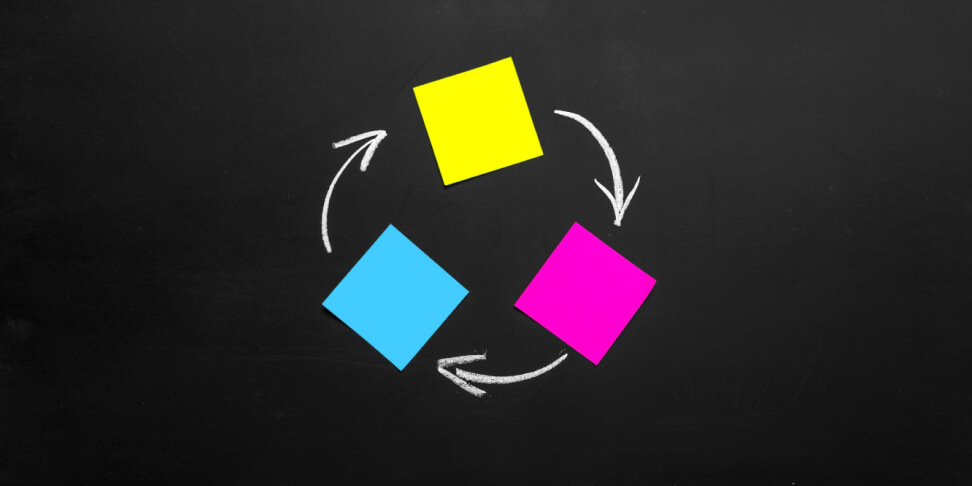
Autor: 05.06.2023
Java - type conversion and type casting
When it comes to converting one Java data type into another, we got two basic operations: type casting and type conversion. Let’s see how they work!
Type Conversion
Conversion is about turning one type into another one. The illustration below shows possible conversions in Java. To make things easier we can hint that conversion occurs when we move from a type that occupies less memory to a type that occupies more memory (or they occupy the same amount of memory).
Simple examples:
byte (1 byte) to short (2 bytes)
short (2 bytes) to int (4 bytes)
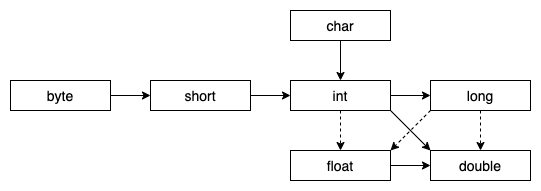
Solid arrows indicate conversions that do not cause data loss. Dashed arrows indicate conversions that may cause loss of some data.
An example of data loss.
The large integer 123456789 is too huge to fit into the float type. When we convert this number to a float type, we will lose precision. Take a look:
public class Main {
public static void main(String[] args) {
int number = 123456789;
float numberF = number;
System.out.println("number: " + number);
System.out.println("numberF: " + numberF);
}
}
Result:
number: 123456789
numberF: 1.23456792E8
Basic rules of conversion
Combining variables of different types involves some rules that you should know:
- if any number is of type double, the other number will also be converted to double,
- otherwise, if any number is of float type, the second number is also converted to float type,
- otherwise, if any number is of type long, the second number is also converted to type long,
- otherwise both numbers will be converted to int type.
Example of combining float type variables with int type variables:
public class Main {
public static void main(String[] args) {
float pi = 3.14f;
int r = 2;
float area = 2 * pi * r;
System.out.println("circle area: " + area);
}
}
Result:
circle area: 12.56
As you can see it works, but you have to be aware of what you are doing.
Type Casting
Converting a variable of type int to double is possible. But what would happen if we tried to do it the other way around? Look at the code below and see the result:
public class Main {
public static void main(String[] args) {
double variableA = 10.1;
int result = variableA;
}
}
Result:
incompatible types: possible lossy conversion from double to int
An error appeared which is, "incompatible data types".
We can apply a forceful solution here, which is casting. The compiler warns about the loss of data, but you can ignore it and make a cast. How to do that? To perform a cast you just have to put the type name in the parentheses before the name of the variable to be casted. The situation is shown below.
public class Main {
public static void main(String[] args) {
double variableA = 10.1;
int result = (int)variableA;
}
}
As a result of the cast, the result variable will have the value 10 because casting a floating point number to an integer removes its fractional part. Be aware of your actions. The compiler warned against such a situation.
Summary
Type Conversion and Type Casting are pretty important parts of the Java language. Be aware of some pitfalls that we mentioned in this short article!
If you want to learn Java from the basic level and acquire the skills needed for a Junior Java Developer position, start your learning journey with the Career Path. The learning concludes with an exam that qualifies you to use the Certificate of Completion.