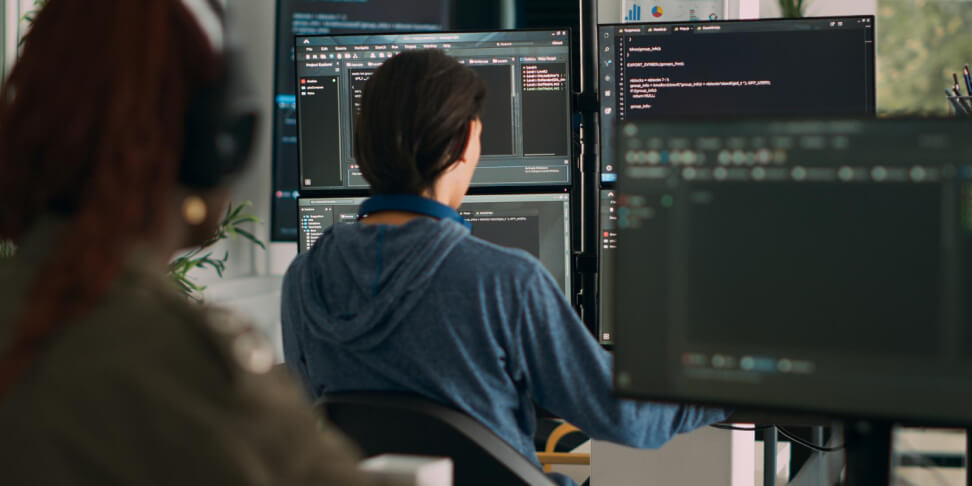
Autor: 03.04.2024
Programming - A Complete Guide for Beginners
The skill of programming opens up unlimited possibilities for creation, development, and innovation. In this comprehensive guide, you will learn the foundations of programming, the most important programming concepts, and the key techniques of a programmer's work. You will also discover how to start learning programming. This guide is intended for beginners.
1. Basics of programming
Programming is nothing more than the art of writing instructions that a computer can understand and execute. As you probably know, there are various programming languages. They differ in applications and technical details. Each language has its syntax, which defines a set of available rules. Therefore, learning programming is somewhat similar to learning a foreign language: you need to master the most important words and rules of expression at the beginning.
Most popular languages are based on the same fundamentals. And it is precisely from these fundamental concepts that we will begin our guide.
Variables, data types, and operators
Variables are containers for storing values. An example of a variable looks like this:
x = 10
We have a variable named x, which stores the value 10. Thanks to this construction, we can easily refer to the value and perform various operations on it.
There are also different data types. Variables can store integers, Boolean values (true/false), text, etc.
In programming languages, we often use so-called operators. These are special characters with which we perform various operations. Examples include:
- == (double equal sign) is an operator that checks if two values are equal
- (plus) is an operator that adds two values
- (greater than sign) is an operator that checks if the value on the left is greater than the value on the right.
Conditional statements and loops
In programming, we often use conditional statements - they allow programs to make decisions. A conditional statement works on a simple principle: if a condition is met, perform action A; if the condition is not met, perform action B. Here's an example of a simple conditional statement:
if x == 10 {
print(“Condition met”)
}
This statement will display the message "Condition met" only if the value of the variable x is equal to 10 (pay attention to the use of the comparison operator!).
Computers excel at performing repetitive tasks. In programs, such tasks can be realized using loops. It's a special construct that repeats the action specified by us many times:
for (let x = 0; x < 5; x++) {
print(x)
}
The above loop will generate values from 0 to 4. Similarly, you could create a loop that works thousands or even millions of times.
Functions and procedures
In programming, we often use functions and procedures. We have described the differences between functions and procedures in this article. A function is a program fragment that we can use multiple times - this makes the programmer's work easier. Here's an example function:
my_function(a, b) {
return a + b
}
The above function takes two parameters (a and b) and returns their sum. You define such a function once, and then you can use it many times.
2. Data structures and algorithms
Data structures
Data structures are an important part of programming. This is the way we store sets of data in a program. We have various popular structures: list, queue, stack, tree. Each of them has its advantages and disadvantages. An experienced programmer knows which data structure will be optimal in a given situation.
Algorithms
An algorithm is a set of steps leading to a solution to a problem. Imagine you want to add two numbers. Let's write it down in steps:
- Get the value of number a and b
- Add the value of a and b
- Display the result on the screen
In real programs, algorithms are, of course, more complex. Examples: sorting algorithms, searching algorithms, algorithms recognizing objects in images, algorithms calculating an account balance, etc.
One of the key features of a good programmer is knowledge of the key principles of algorithm design . This is a fundamental skill in this industry.
Graphs and graph algorithms
You may be familiar with the concept of a graph from mathematics. Such graphs and graph algorithms are an important part of modern programs. Thanks to them, we can plan optimal routes, optimize logistics, or store related information.
Recursion
You may sometimes come across the concept of recursion. A recursive function is a special function that calls itself. In some situations, this is a good way to solve a programming problem optimally. You can find more about recursion here.
3. Programming Paradigms
Programming paradigm is the way we approach the design and analysis of a program. It could be said that it's a certain style of writing a program.
Imperative and Declarative Programming
In simplified terms, we can divide programming into two main groups: imperative programming and declarative programming. Imperative programming resembles guiding the computer step by step on what to do. On the other hand, in declarative programming, we focus more on the result, on what we want to achieve, without delving into detailed steps.
Popular Programming Paradigms
In object-oriented programming, , the entire program structure is built with classes and objects. This approach allows easy representation of structures from the world around us. Imagine an object Article. It has certain properties: title, author, content. It also has certain behaviors: publication, update, etc. This is an example of object-oriented thinking.
Also popular is the functional approach to programming. Instead of classes and objects, we have functions, which are small, independent blocks of code. This approach allows building the program structure from smaller "blocks".
There are also other paradigms, such as event-driven programming. It is widely used in applications that need to respond appropriately to events triggered by the user.
4. User Interaction
Even the simplest program has some input and output. Thanks to this, it can accept some data, perform operations on them, and return the result.
Graphical User Interfaces
Programs can also be very complex and offer unlimited interaction with the user. Building a graphical user interface (GUI) for such a program is a significant challenge. Many popular programming languages have tools that, to some extent, facilitate this task. Often, they take the form of so-called libraries or frameworks that offer ready-to-use user interface elements.
5. Databases
Basics of Databases and SQL
Most programs need to store data somewhere. A database is simply an organized set of such data. We have different types of databases: relational, graph, NoSQL, etc. Each of them has its pros, cons, and typical uses.
The most common ones are relational databases, based on the SQL language. Most beginner programmers start their journey with databases from them.
Basic Data Operations
With SQL, we can write so-called queries to the database. We distinguish four basic operations:
- Create
- Read
- Update
- Delete
These four operations create an acronym known as CRUD
6. Software Development
A good programmer knows techniques that allow working in an organized way. They also know techniques that will help them create error-free programs.
Debugging and Testing
Debugging is the process of finding and fixing errors in a program. Testing, on the other hand, improves the quality of the code and ensures that the program works as expected. There are different testing methodologies used at different stages of the program development. Some make sense in the early stages of work, while others are used to test the finished program.
Project Management
Programming is often teamwork. Some programs are so complex that their development requires the work of hundreds or even thousands of people. In such a situation, methodologies and tools for project management are of enormous importance.
Documentation and Comments
The program code must be readable for the computer. But it should also be readable for humans. A good programmer takes care of proper documentation of the code they have written. They regularly write comments that explain individual parts of the program code. In the case of teamwork, this is simply essential. The code should be clear and understandable.
7. Security and Optimization
Creating Secure Software
Our modern life relies on applications and digital services. Therefore, security is crucial. Wherever we deal with sensitive data, techniques for creating secure applications become particularly important. There are some fundamental principles:
- Using encrypted data transmission based on HTTPS
- Ensuring password security
- Applying practices to increase program resilience to attacks
- Regularly updating software
A good application should be efficient, fast, and reliable. Therefore, a good programmer should know code optimization techniques. They should know how to write efficient algorithms and ensure uninterrupted access to the program. It's not an art to write a program that somehow works. The art lies in making it work fast, efficiently, and reliably.
8. Tools and Development Environments
Besides language proficiency, a programmer also needs certain tools. For coding, we need an editor. There are various solutions available on the market: simple and more complex, free and paid. The term IDE, Integrated Development Environment , is often used. It's a complete set of tools for writing programs: editor, debugger, compiler.
Version Control System
Complex programming projects often consist of hundreds of files. Thousands of different changes are made to these files. To manage this, a special tool is needed, known as a version control system. The most popular tool of this type is Git
Virtual Environments and Containerization
How to ensure that every programmer in the team has the same working environment? The solution is containerization. Thanks to it, the application can be packed together with the environment necessary for its operation.
9. Specializations in Programming
In programming, we have different specializations and different skills required in each specialization.
Web Development
Web development involves creating applications that operate on a server and in a web browser. Sometimes, such a programmer is referred to as a Web Developer . This specialization is often divided into two niches:
- Back-end Developer: a programmer who writes applications that run on the server.
- Front-end Developer: a programmer who writes applications that run in the browser. This refers to what is directly visible to the user.
Popular programming languages in web development include HTML, CSS, JavaScript, C#, and Java.
Mobile Development
This field involves creating applications for phones with Android and iOS systems. Popular languages in this field include Kotlin, Java, and Swift.
Data Analysis and Machine Learning
The popularity of this programming sector has literally exploded in recent years. We're talking about areas such as Machine Learning, data processing, and writing AI algorithms. Python language is often used in this field.
Game Development
Another popular niche is game development. Games can be quite demanding in terms of programming skills. Therefore, this is a field where you can find many enthusiasts fascinated by their work. There are also certain tools that facilitate game development.
11. How to Learn Programming
Programming is a complex field, requiring considerable knowledge. Therefore, learning programming requires a good plan. It cannot be mastered overnight. A good plan and consistency in action are the keys to success. You must have patience and determination.
Methods of Learning
There are various ways to learn programming: textbooks, videos, studies, courses, etc. There are no perfect solutions because the effectiveness of a given method depends on your capabilities and preferences. Think about what learning method suits you best.
Practice and Exercises
Programming is a very practical field, where your real skills are of great importance. In short, the learning plan looks like this:
- Master the basics of a programming language
- Start practicing and writing simple programs
- Choose a specialization that interests you
- Learn the most important tools and technologies used in that specialization
- Practice even more
- Start preparing for your first job interview.
As you can see, exercising your skills is very important. From the beginning, try to solve programming problems: write simple algorithms and create simple programs. Continuously think about how you can practice and expand your knowledge.
And most importantly - don't give up! Treat unsuccessful job interviews as an opportunity to learn. Fill in the gaps in your knowledge and try again. Good luck!